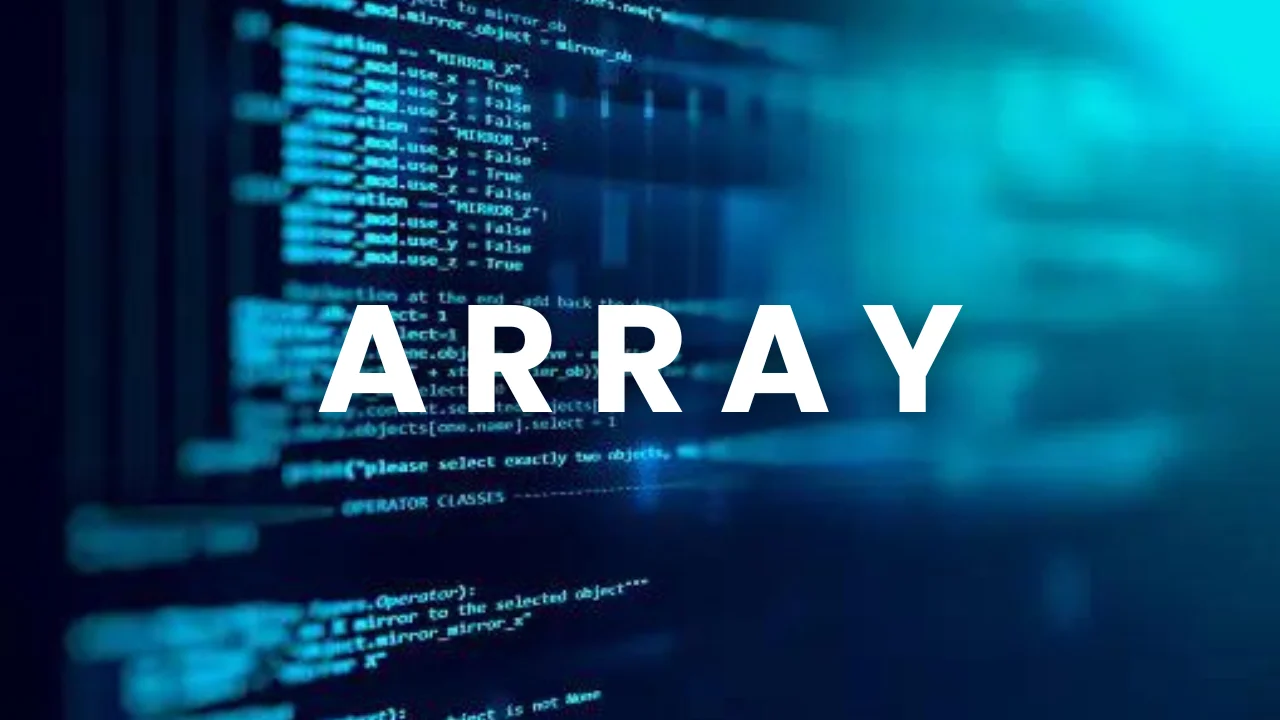
Arrays in C
In C, an array is a data structure that stores a fixed-size sequential collection of elements of the same type. Each element in the array occupies a contiguous memory location, and they are accessed by their position, known as the index. Arrays are particularly useful when dealing with a collection of data elements of the same type that need to be stored and accessed efficiently.
Arrays are declared by specifying the data type of the elements they will hold and the number of elements. For example : declares an array of integers with 5 elements.
int numbers[5];
Arrays can be initialized at the time of declaration or later using braces {}. For example : initializes an array of integers with specific values.
int numbers[5] = {1, 2, 3, 4, 5};
Elements in an array are accessed using an index. Array indices start from 0 and go up to (size - 1). For example: numbers[0] accesses the first element, numbers[1] accesses the second element, and so on.
Output :
Array elements: 10 20 30 40 50
Explanation :
- We declare an array of integers named numbers with a size of 5.
- We initialize each element of the array with values 10, 20, 30, 40, and 50, respectively.
- We then print out all the elements of the array using a loop, iterating through each element and printing it.
Output :
sum=291
average=48.500000
Output :
Max=91
Output :
Enter a range=4
Enter number=1
Enter number=2
Enter number=3
Enter number=4
After reverse=4 3 2 1
In Conclusion, arrays play a fundamental role in C programming by providing a mechanism for efficient data storage, manipulation, and access. Their importance lies in their ability to simplify programming tasks, improve memory management, and enhance code efficiency and readability.