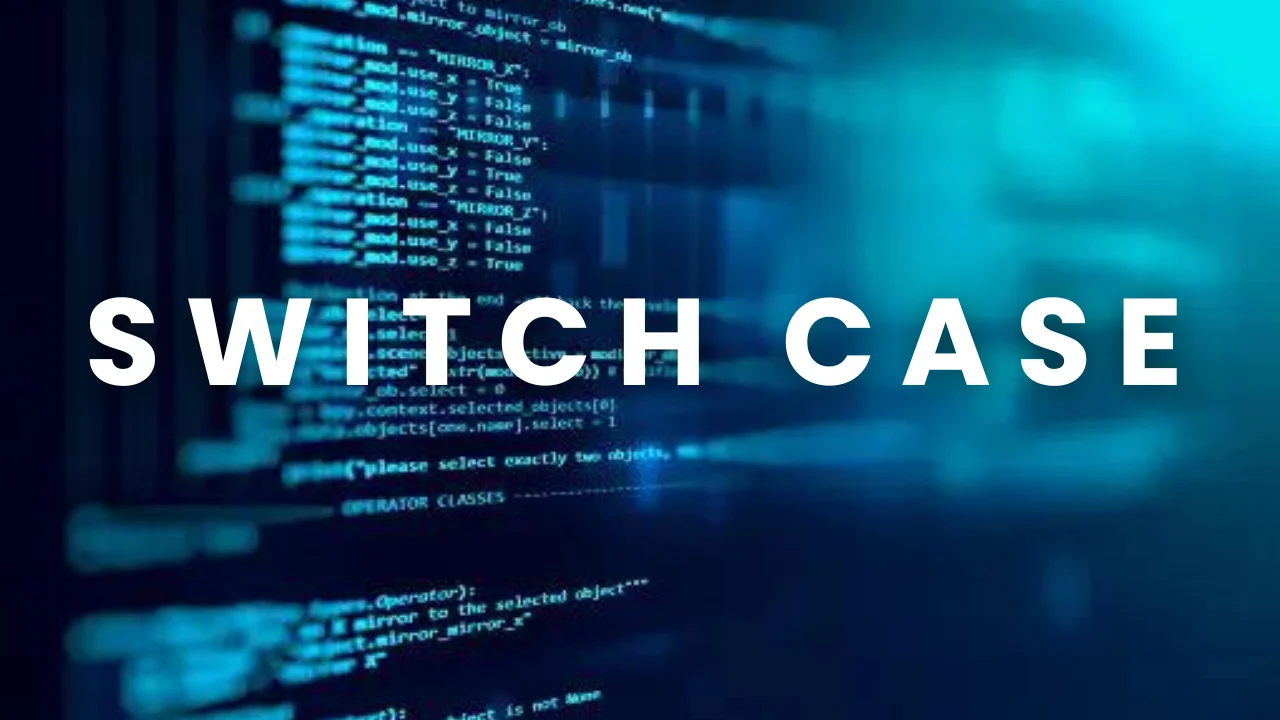
switch case in C
The switch statement in C is a control structure used to execute one block of code among many based on the value of an expression. It allows you to compare the value of a variable or expression against a series of constants and execute different blocks of code depending on which constant matches the value.
switch (expression) {
case constant1:
// code to be executed if expression equals constant1
break;
case constant2:
// code to be executed if expression equals constant2
break;
// you can have any number of case statements
default:
// code to be executed if expression doesn't match any case
}
expression : The variable or expression whose value is compared against the case constants.
case constant : A constant value that the expression is compared to.
break : Exits the switch statement. If omitted, execution will continue to the next case (fall-through).
default : Optional. Executes if no case matches the expression.
Explanation :
- The variable day is set to 3.
- The switch statement compares day to each case.
- When day is 3, the code in case 3: executes, printing "Wednesday".
- The break statement prevents the execution from falling through to the next cases.
- If day were not between 1 and 7, the default case would execute, printing "Invalid day".
Output :
Enter month no :5
31 days
Output :
1.fah to cel
2.cel to fah
enter choice:1
enter fah val:200
297
Output :
VIBGYOR
Enter colour : R
red
The switch statement in C is a powerful control structure that enhances code readability, maintainability, and efficiency. It is particularly useful when dealing with a variable that can take on multiple discrete values. By centralizing all related conditions, it provides a clear and organized way to handle complex branching logic.