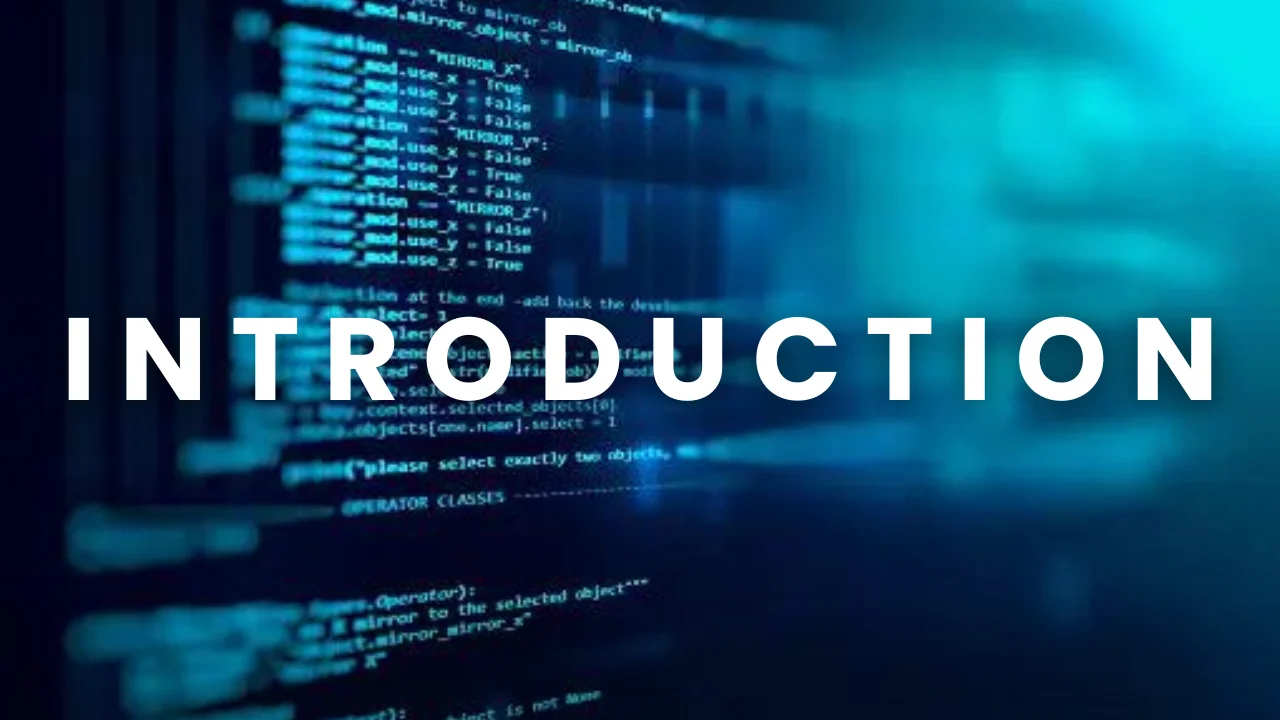
Introduction to C Programming
C is a powerful and versatile programming language developed by Dennis Ritchie at Bell Labs in the early 1970s. It is a procedural, general-purpose language that has greatly influenced many other programming languages due to its efficiency and flexibility.
Procedural Language : C follows a procedural programming paradigm, where the program is structured as a series of functions that manipulate data.
Portability : C programs can be easily ported across different platforms with minimal changes, thanks to its standardized specifications and widespread compiler support.
Efficiency : C provides low-level access to memory and hardware, allowing for efficient code execution and optimization.
Modularity : C supports modular programming through the use of functions and libraries, allowing for code reuse and easier maintenance.
Static Typing : C is statically typed, meaning that variable types are determined at compile time, which helps catch errors early in the development process.
C syntax is relatively simple compared to other languages.
It uses a combination of keywords, identifiers, operators, and punctuation to form statements and expressions.
Statements are terminated by a semicolon (';''), and blocks of code are enclosed within curly braces ('{}'').
C has several basic data types such as :
int : Used to store integer values. Typically 4 bytes in size.
char : Used to store single characters. 1 byte in size.
float : Used to store single-precision floating-point numbers. Typically 4 bytes in size.
double : Used to store double-precision floating-point numbers. Typically 8 bytes in size.
Variables are used to store data values and must be declared before use, specifying their data type. Constants are values that do not change during program execution and are declared using the 'const' keyword.
C supports various operators such as arithmetic operators ('+', '-', '*', '/', '%''), relational operators ('==', '!=', '<', ''>', '<=', ''>=''), logical operators ('&&', '||', '!''), and assignment operators ('=', '+=', '-='').
C provides control flow structures like 'if', 'else', 'switch', 'while', 'do-while', and 'for' loops for decision-making and repetition.
C comes with a standard library ('<stdio.h>', '<stdlib.h>', '<math.h>', etc.) providing functions for input/output, memory allocation, mathematical operations, string manipulation, and more.
Output :
Hello, PBA INSTITUTE
Here's what this code does :
#include <stdio.h> : </stdio.h> This line includes the standard input-output library, allowing you to use functions like printf().
int main() { ... } : This is the main function, where program execution begins. The { ... } denotes a block of code.
printf("Hello, PBA INSTITUTE"); : This line prints "Hello, PBA INSTITUTE" to the console. printf() is a function used to print formatted output.
Output :
sum=16
Output :
Area of square=36
Overall, C remains a fundamental language in the field of computer programming, serving as a solid foundation for learning other languages and understanding computer systems at a deeper level. To understand C thoroughly, it's essential to practice writing code, experiment with different constructs, and gradually build proficiency through hands-on experience. Additionally, studying sample code, reading documentation, and referring to reputable learning resources can further aid comprehension.