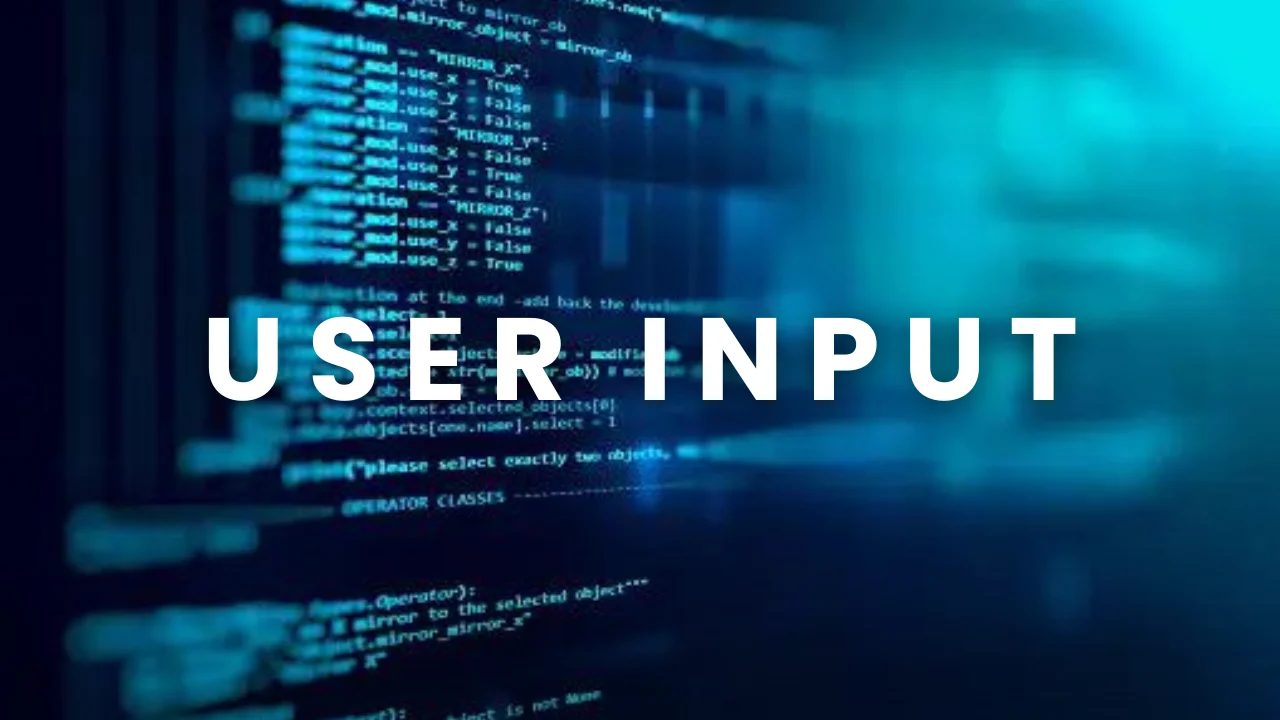
User Input in C
Understanding user input in C involves grasping the different functions available to read data from the user and how to properly handle various types of input. Here’s an in-depth look at handling user input in C.
The scanf function is used for reading formatted input. It reads data from the standard input stream stdin based on the format specifiers provided. Common format specifiers include %d for integers, %f for floats, %c for characters, and %s for strings.
scanf(format, &variable);
Example :
int number;
scanf("%d", &number);
Output :
Enter a number: 5
You entered: 5
Output :
Enter the marks in physics: 70
Enter the marks in chemistry : 80
Enter the marks in biology : 90
Total marks obtained=240.000000
Average marks obtained=80.000000
Output :
Enter the value of d1 :8
Enter the value of d2 :4
Area=16
Output :
Enter the value of b:5
Enter the value of s:10
Enter the value of h:15
Base area=25
Surface Area=125
Volume=125
User input plays a crucial role in programming and software development by enabling interactivity, customization, flexibility, decision-making, data acquisition, error detection, collaboration, and innovation. Incorporating user input effectively enhances user experiences, drives user engagement, and contributes to the success of software products and services.