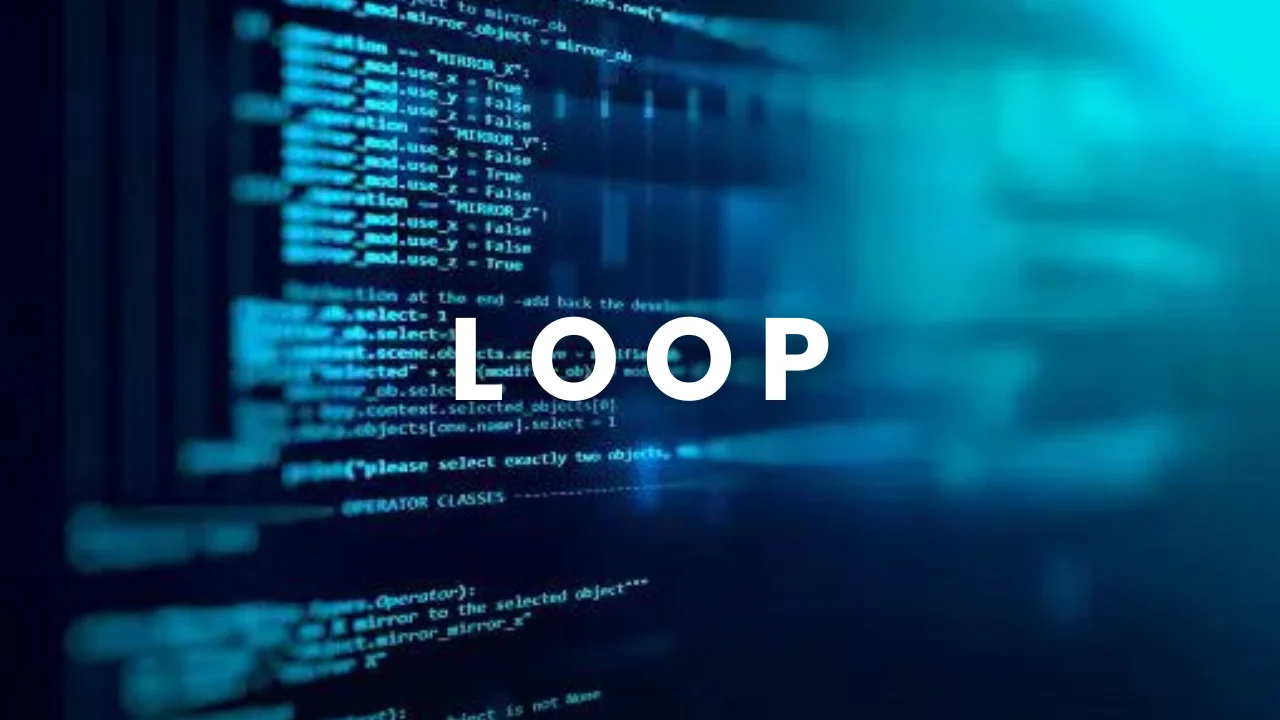
Loops in C
Loops are a fundamental part of the C programming language, enabling the execution of a block of code multiple times. This functionality is crucial for performing repetitive tasks, handling large data sets, and automating operations.
A loop is a control structure that allows you to execute a block of code repeatedly based on a condition. This is useful for performing repetitive tasks, iterating over data structures, and handling dynamic conditions.
Initialization : Setting up a starting point for the loop.
Condition : A logical expression that is evaluated before each iteration. If the condition is true, the loop body is executed; if false, the loop terminates.
Increment/Decrement : Updating the loop control variable to eventually meet the termination condition.
1. for Loop :
Ideal for scenarios where you know the exact number of iterations in advance.
2. while Loop :
Best for cases where the number of iterations isn't predetermined and the loop should continue based on a condition.
3. do...while Loop :
Similar to the while loop but guarantees that the code block executes at least once.
4. Nested Loops :
Loops can be nested to handle more complex tasks, such as iterating over multi-dimensional arrays.
Loops are a fundamental construct in C programming, and their importance is significant for several reasons:
Efficiency and Performance :
Using loops can significantly enhance the performance of a program. Instead of manually writing code for repetitive tasks, loops can automate these tasks, reducing the amount of code and minimizing the potential for errors.
Handling Large Data Sets :
When dealing with large data sets, such as arrays, lists, or files, loops are essential for processing each element or record.
Automation and Simplification :
Loops automate repetitive tasks, simplifying complex operations. For instance, initializing elements of an array, checking each value, or applying a function to a series of inputs can be easily managed with loops.
Dynamic Conditions :
Loops can handle scenarios where the number of iterations is determined at runtime.
Maintainability and Scalability :
By using loops, you create code that is easier to maintain and scale. If the number of iterations needs to change, you simply update the loop's condition or range, rather than rewriting large sections of code.
Reduction of Code Duplication :
Loops help in reducing code duplication by encapsulating repetitive logic within a single block of code. This not only shortens the code but also makes it easier to debug and modify.
Loops are crucial for effective programming in C because they enable automation of repetitive tasks, efficient handling of large data sets, dynamic and flexible conditions, and reduced code duplication. Mastery of loops leads to writing more maintainable, scalable, and efficient code.