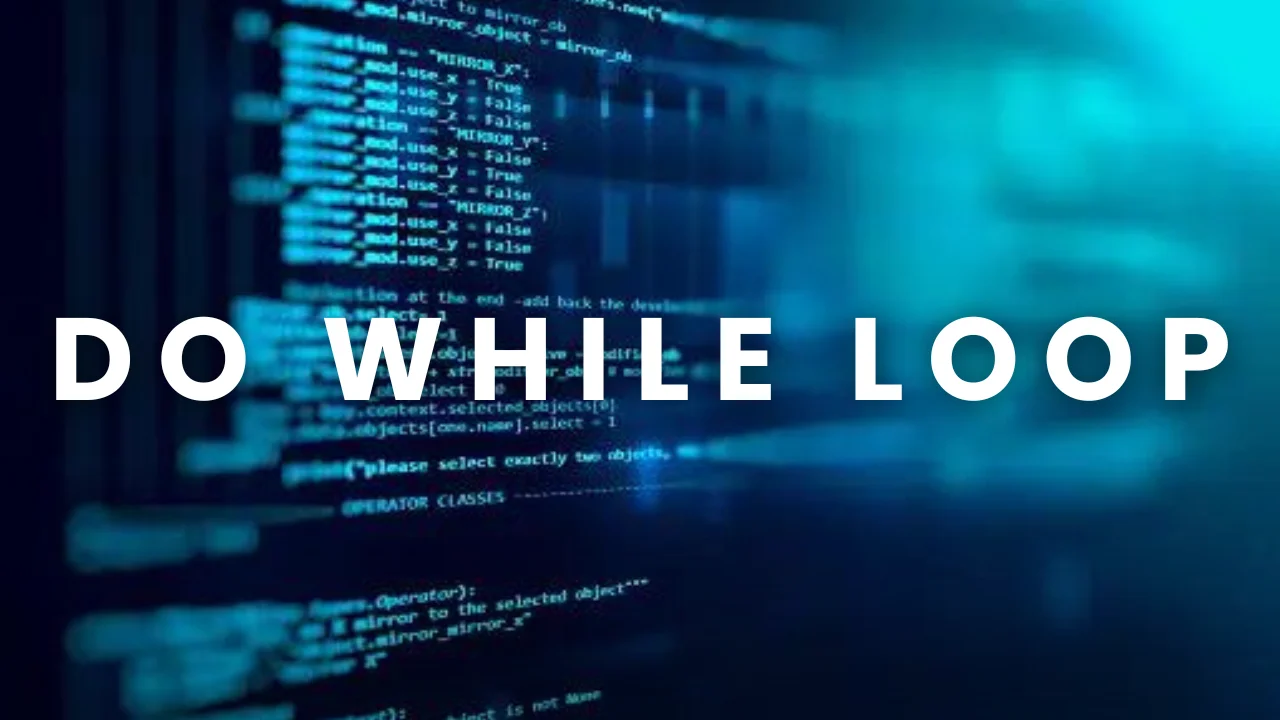
do-while loop
A do-while loop in C is a control flow statement that repeatedly executes a block of code as long as a specified condition evaluates to true.
The syntax of a do-while loop is as follows :
do {
// Code to be executed
} while (condition);
1. The code inside the do block is executed first.
2. After executing the code in the do block, the condition specified in the while is evaluated.
3. If the condition evaluates to true, the loop will execute the code in the do block again.
4. This process repeats until the condition evaluates to false.
A do...while loop is particularly useful when you need the code block to execute at least once regardless of whether the condition is initially true or false. Common scenarios include :
- Presenting a menu to a user at least once.
- Repeating a block of code until a specific user input is received.
Output :
i = 1
i = 2
i = 3
i = 4
i = 5
Explanation of the Example :
Initialization : The variable i is initialized to 1.
Execution : The do block is executed, printing the value of i and then incrementing i by 1.
Condition Check : After the do block, the condition i <= 5 is checked.
Repetition : If the condition is true, the loop executes the do block again. If the condition is false, the loop terminates.
The primary difference between a while loop and a do-while loop is the timing of the condition check :
while Loop : Checks the condition before executing the code block. If the condition is false at the beginning, the code block may not execute at all.
do-while Loop : Executes the code block first and then checks the condition, ensuring that the code block is executed at least once.
Output :
Enter the number=123
Sum of digit=6
Output :
Enter a number=9
Neon number
Output :
Enter the number=153
Armstrong number
The do-while loop in C is an essential control structure that provides a straightforward way to ensure a block of code runs at least once before any condition is tested. Its importance lies in scenarios requiring minimum one-time execution, user input validation, menu-driven programs, and cases where the condition depends on an initial operation. By using do...while loops appropriately, programmers can write more effective, readable, and maintainable code.