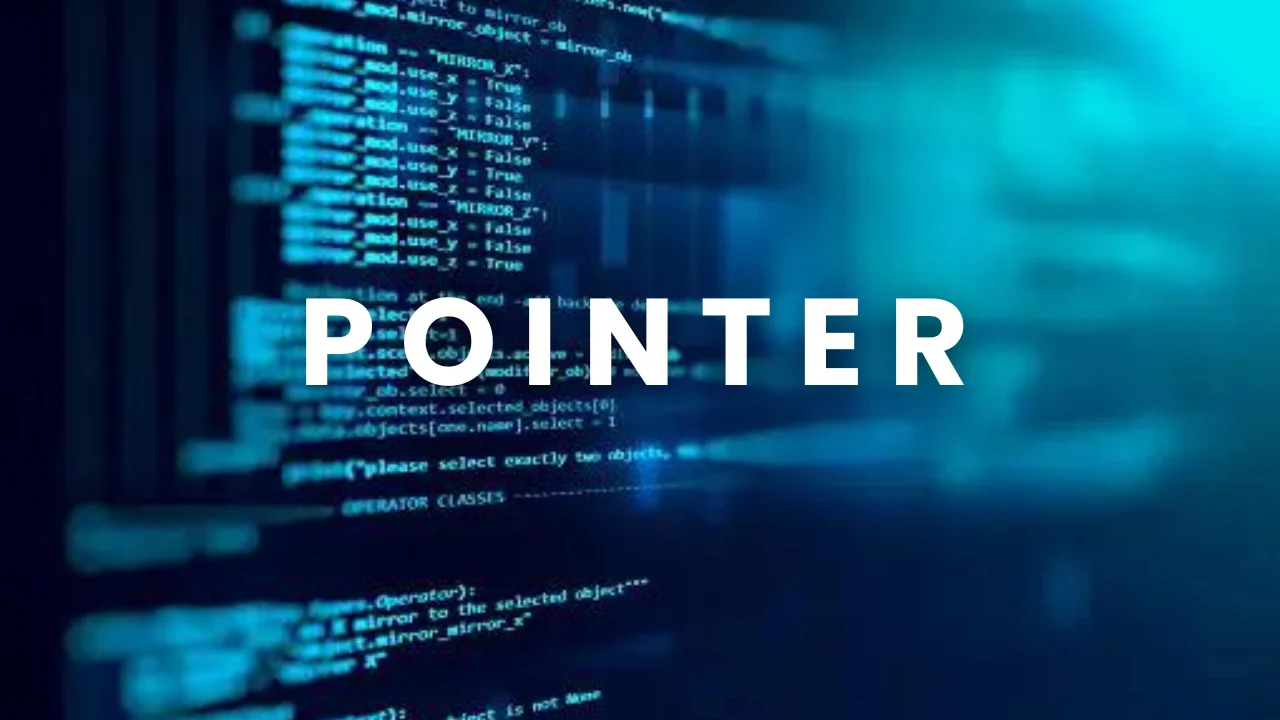
Pointers in C
A pointer is a variable that stores the memory address of another variable. Instead of holding the actual value of the variable, a pointer holds the location of where the variable is stored in memory.
To declare a pointer, you use an asterisk ('*') followed by the name of the pointer variable. For example, 'int *ptr;' declares a pointer 'ptr' that can point to an integer.
Pointers should be initialized to point to valid memory locations. This is typically done using the address-of operator ('&') with the variable whose address you want the pointer to hold.
For example : 'int x = 10; int *ptr = &x;' initializes 'ptr' to point to the integer variable 'x'.
To access the value pointed to by a pointer, you use the dereference operator ('*').
For example : 'int y = *ptr;'' assigns the value of 'x' (which 'ptr' points to) to 'y'.
Pointers in C can be incremented and decremented to move through memory locations.
For example : if 'ptr' points to an integer, 'ptr++' will make 'ptr' point to the next integer location in memory.
Arrays in C are essentially pointers to the first element of the array.
For example : if 'int arr[5];' declares an array of 5 integers, then 'arr' can be used as a pointer to the first element ('&arr[0]').
Pointers are often used in functions to pass variables by reference. This allows functions to modify the original variables passed into them. This is done by passing the pointer to the variable instead of the variable itself.
Pointers can point to other pointers. This is useful for tasks like dynamically allocating arrays of pointers, creating multi-dimensional arrays dynamically, and for passing pointers by reference to functions.
Pointers are essential for dynamic memory allocation in C using functions like 'malloc', 'calloc', 'realloc', and 'free'. These functions allocate and deallocate memory at runtime, and pointers are used to access and manage this memory.
Output :
Value of x: 10
Address of x: 000000000062FE14
Value pointed to by ptr: 10
Explanation :
- 'int x = 10;' : Declares an integer variable 'x' and initializes it to 10.
- 'int *ptr = &x;' : Declares a pointer variable 'ptr' that points to an integer ('int *''). It is initialized with the address of 'x' using the address-of operator '&'.
- 'printf("Value of x: %d\n", x);' : Prints the value of 'x'.
- 'printf("Address of x: %p\n", (void *)&x);' : Prints the memory address of 'x' in hexadecimal format. The '(void *)'' cast is used to ensure proper type matching for the '%p' format specifier.
- 'printf("Value pointed to by ptr: %d\n", *ptr);' : Dereferences 'ptr' using '*ptr' to access and print the value of 'x'.
Output :
Enter the two numbers=10
15
The value of a after swap=15
The value of b after swap=10
Output :
Enter the range=3
Enter the array elements=1
2
3
sum=6
Output :
Enter the string=PBA
Length=3
Null Pointers : Pointers can be explicitly set to 'NULL' or '0' to indicate they are ,not pointing to any valid memory location.
Dangling Pointers : These occur when a pointer continues to point to a memory location that has been deallocated.
Memory Leaks : Failure to deallocate dynamically allocated memory can lead to memory leaks.
Pointers in C are fundamental to efficient memory management, dynamic data structures, function parameter passing, low-level programming tasks, and interacting with hardware. They empower programmers to write optimized, flexible, and powerful code, making C a preferred choice for system-level programming and performance-critical applications.