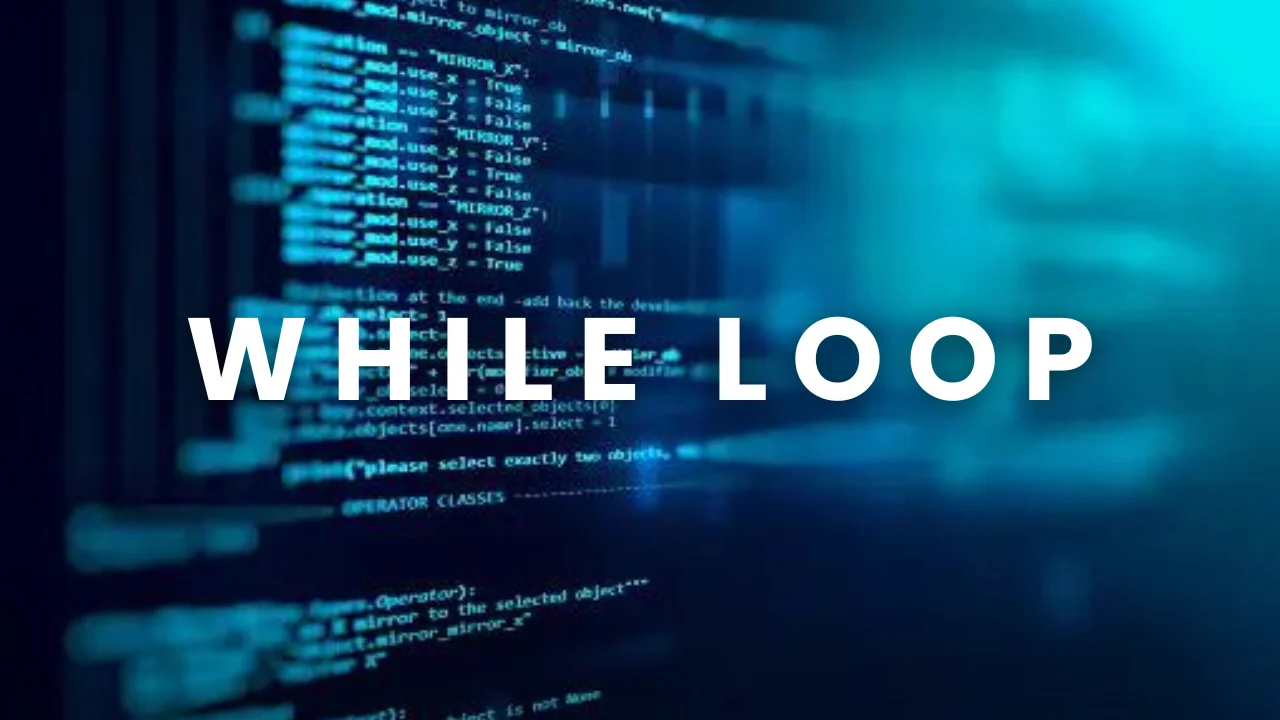
While Loop in C
A while loop in C is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The loop continues to execute the block of code as long as the condition evaluates to true. It is particularly useful when the number of iterations is not known before entering the loop and depends on a condition being met during execution.
while (condition) {
Condition : An expression evaluated before each iteration. If it is true, the loop body is executed. If it is false, the loop terminates.
// Code to be executed
}
loop body : The block of code that is executed as long as the condition is true.
Here's an example that uses a while loop to print numbers from 0 to 4 :
Output :
i = 0
i = 1
i = 2
i = 3
i = 4
Output :
Enter a no:215
The sum of digits=8
Output :
Enter the no:1234
length=4
Output :
Enter the no:9
Neon no
break : Terminates the loop immediately.
Output :
i = 0
i = 1
i = 2
i = 3
i = 4
continue : Skips the rest of the loop body and proceeds with the next iteration.
Output :
i = 1
i = 3
i = 5
i = 7
i = 9
The while loop in C is an essential control flow statement that provides several key benefits and plays a crucial role in various programming scenarios. Here are some of the reasons why the while loop is important in C :
Flexibility in Loop Control :
The while loop allows for flexible iteration control based on dynamic conditions.
Pre-Test Loop Structure :
As a pre-test loop, the while loop checks the condition before executing the loop body.
Handling Variable Iterations :
While loops are particularly useful for scenarios where the number of iterations isn't fixed or known in advance. They allow for iteration until a certain condition is met, making them suitable for a variety of use cases.
Resource Management :
In systems programming and resource management, while loops can be used to wait for resources to become available or to perform cleanup tasks
Error Handling and Validation :
While loops are often used for input validation and error handling, ensuring that the program continues to prompt the user or reprocess data until valid input is received.
The while loop is a versatile and powerful construct in C programming. Its importance lies in its flexibility, ability to handle variable iterations, suitability for dynamic conditions, and role in a wide range of programming scenarios, from input validation to complex algorithm implementation. By providing a straightforward mechanism for repeated execution based on a condition, while loops are fundamental to writing efficient, readable, and maintainable code in C.