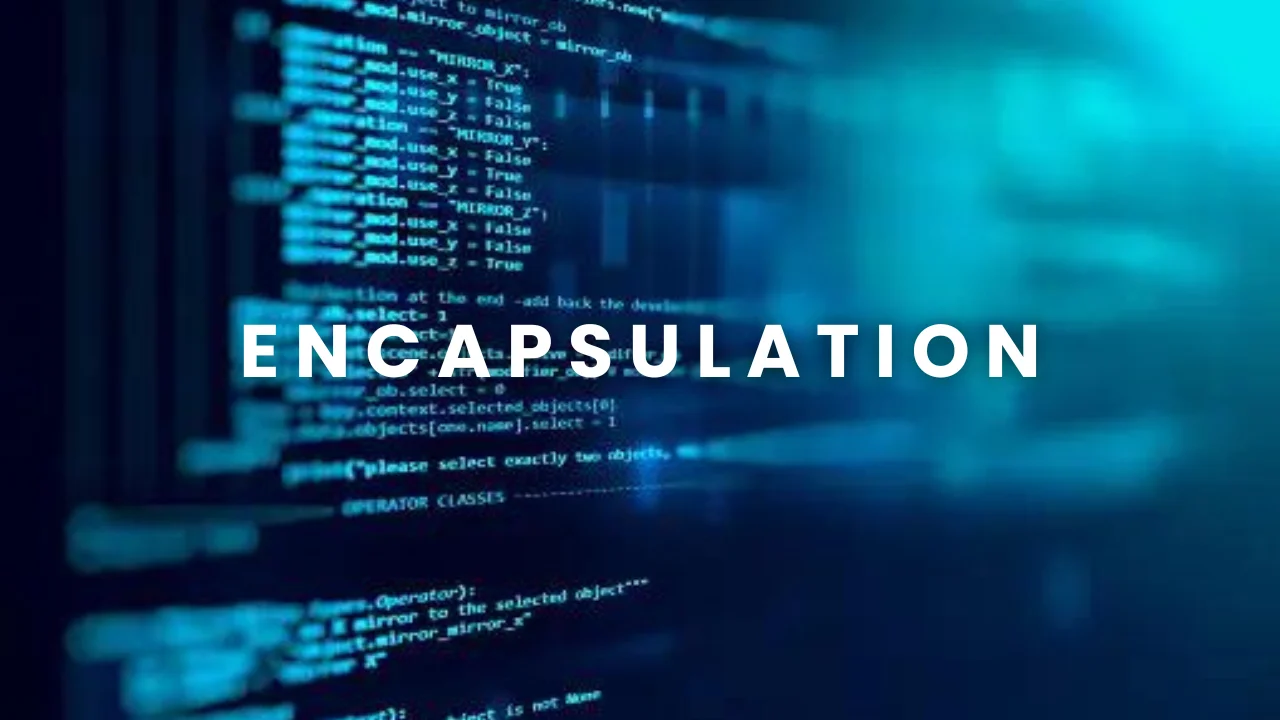
Understanding Encapsulation:
Encapsulation in Java is one of the fundamental concepts of object-oriented programming (OOP). It refers to the bundling of data and methods that operate on the data into a single unit or class, and then controlling access to that unit. The main idea behind encapsulation is to hide the internal state of an object and only expose the necessary functionalities via public methods.
Here's how encapsulation works in Java:
1.Private Members:Encapsulation involves declaring the variables of a class as private, meaning they can only be accessed within the same class. This prevents direct access or modification of the class's internal state from outside the class.
2.Public Methods:To allow controlled access to the private variables, public methods are created within the class. These methods, also known as getter and setter methods, provide a way for other classes to interact with the private variables indirectly.
3.Getter and Setter Methods:Getter methods are used to retrieve the values of private variables, while setter methods are used to modify the values of private variables. These methods can include additional logic for validation or computation.
Q-1 Implement a class Carton as per following documentation:
Class Carton // A class that implements a carboard carton
Constructor:
Carton (double width, double height, double length)
Methods
double volume ()
double area ()
In the current implementation of carton make all the instance variable private. This means that only methods of a carton object can see the object’s data. The object will be immutable if there are no access methods that make changes to this data.Give the public access to methods of Carton. Test your Carton class property.
OUTPUT:
volume:2905.66455
area: 91.68
Encapsulation in Java offers several advantages, which contribute to the overall robustness, maintainability, and flexibility of object-oriented programs. Here are some key advantages:
1.Data Hiding:Encapsulation allows you to hide the implementation details of a class from other objects. By making attributes private and providing public getter and setter methods, you control how data can be accessed and modified from outside the class. This prevents external code from directly accessing sensitive or critical data, thus enhancing security and preventing accidental misuse.
Reusability:Encapsulation supports the concept of reusability by allowing classes to be used by other parts of the program or in different projects without modification. By exposing a clear, well-defined interface (public methods), other developers can use the class without needing to understand its internal workings, as long as the interface remains consistent.
Code Organization: Encapsulation encourages a modular approach to software design. By grouping related attributes and methods into a single class, you create a modular unit that can be easily understood, reused, and modified. This improves code readability, reduces complexity, and makes the codebase more manageable.
Encapsulation in Java promotes data hiding and abstraction by encapsulating data and methods within a class. This ensures controlled access to the object's internal state, enhancing modularity and maintainability. With private instance variables and public methods, encapsulation facilitates flexibility and extensibility while protecting the integrity of the class. Overall, it fosters robust, scalable software development by emphasizing code organization and reusability.