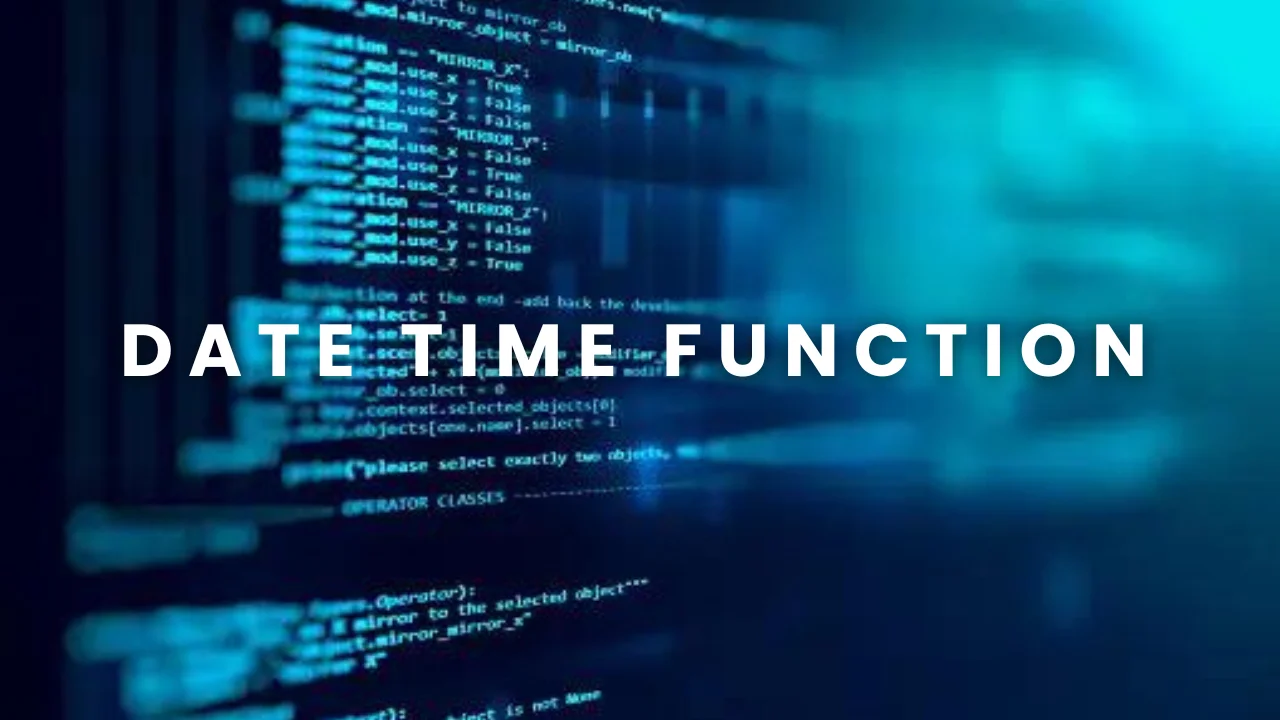
Date Time Functions in Java
Handling dates and times is a crucial aspect of many software applications, ranging from scheduling events to calculating time differences between transactions. Prior to Java 8, Java's standard library provided the 'java.util.Date' class for representing dates and times, but it had several shortcomings, including mutability, lack of thread safety, and limited functionality.
To address these issues and provide a modern, comprehensive API for date and time manipulation, Java 8 introduced the 'java.time package'. This package, also known as the Date-Time API, includes a set of classes to represent dates, times, durations, and periods. It follows the ISO 8601 standard for date and time representation and provides better support for internationalization and time zone handling.
The key classes and interfaces in the java.time package include:
1.Instant:Represents a point in time, typically used for machine-based timing.
2.LocalDate:Represents a date without a time zone, consisting of year, month, and day.
3.LocalTime:Represents a time without a time zone, consisting of hour, minute, second, and fractional seconds.
4.LocalDateTime:Represents a date and time without a time zone.
5.ZonedDateTime:Represents a date and time with a time zone.
6.DateTimeFormatter:Allows formatting and parsing of dates and times.
7.Period:Represents a period of time, such as "2 years and 3 months".
8.Duration: Represents a duration of time, such as "5 hours and 30 minutes"..
9.TemporalAdjusters:Provides methods to adjust dates, such as finding the first day of the month.
10.ChronoUnit:Represents units of time, such as days, hours, minutes, etc.
Question.1 Write a Java program to get the last day of the current month.
Solution
Question.2 Write a Java program to get the dates 10 days before and after today.
OUTPUT:
Current Date: 2024-07-20
10 days before today will be 2024-07-10
10 days after today will be 2024-07-30
1.Write a Java program to create a Date object using the Calendar class.
2.Write a Java program to get the maximum value of the year, month, week, date from the current date of a default calendar.
3.Write a Java program to calculate the first and last day of each week.
In conclusion, Java's java.time package revolutionizes date and time handling with its comprehensive set of classes and methods. Offering immutability, thread safety, and adherence to ISO 8601 standards, it surpasses the limitations of the legacy java.util.Date class. With functionalities like formatting, parsing, and time zone support, it provides a robust solution for modern date and time requirements, making code more readable and maintainable.