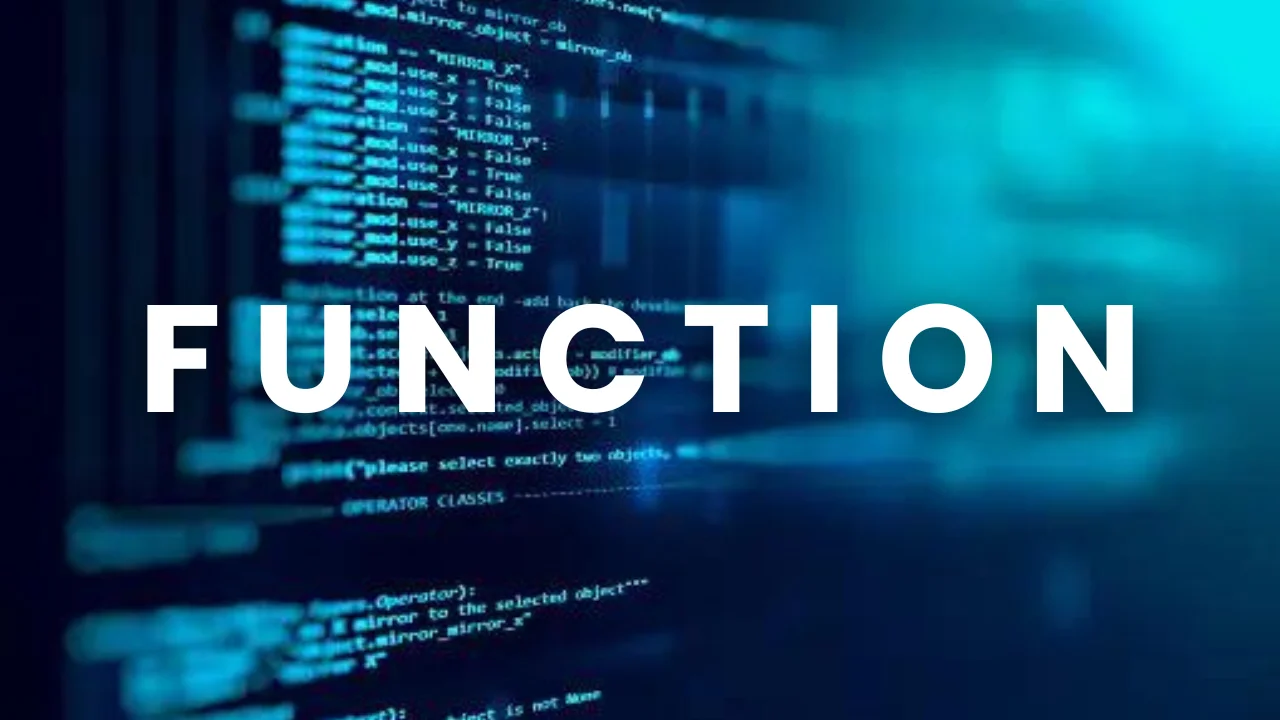
Understanding User-Defined Functions
In Java, a function is typically referred to as a method. A method is a block of code that performs a specific task and can be called from other parts of the program.Here's a breakdown of the full concept of defining a method in Java:
// method body
}
1.Access Modifier:
Specifies the visibility of the method.Like-
i.public:The method can be accessed from any other class.
ii.private:The method can only be accessed within the same class.
iii.protected:The method can be accessed within the same package or by subclasses.
iv.Package-private/default:If no access modifier is specified, it means the method is accessible only within the same package.
2.Return Type: Specifies the data type of the value that the method returns. Use 'void' if the method does not return any value.
3.Method Name:Should be a valid identifier following Java naming conventions.
4.Parameter List:Specifies the data type and name of each parameter that the method accepts. Multiple parameters are separated by commas.
5.Method Body:Contains the code that defines what the method does. It is enclosed within curly braces '{}'.
Question 1.Write a program to input a number. Use a function int Armstrong(int n) to receive the number. The function returns 1 if the number is Armstrong otherwise 0.
OUTPUT:
Enter a number:
153
The output is:
1
Question 2..Write a program to accept a number and check whether the number is prime or not, using the function name check (int n).The function returns 1 if the number is prime otherwise 0.
OUTPUT:
Enter a number:
5
The output is :
1
1. .Write a program to accept a number and check whether number is Even or odd, using the function name void check (int n).
2. Write a program to find the sum of given series to n terms by using function name sum (int).Write the main program to display the sum of the series. S=(1*2) + (2 *3) + (3 *4) + ……………….to n terms.
3.Write a program to accept a number and check whether it is an Automorphic number or not, using function name digits (int n).
User-defined functions in Java offer advantages such as modularity, reusability, and encapsulation. They promote code organization, accept parameters, and return values, enhancing abstraction and efficiency. In conclusion, mastering methods is essential for creating concise, maintainable Java programs with clear structure and functionality.