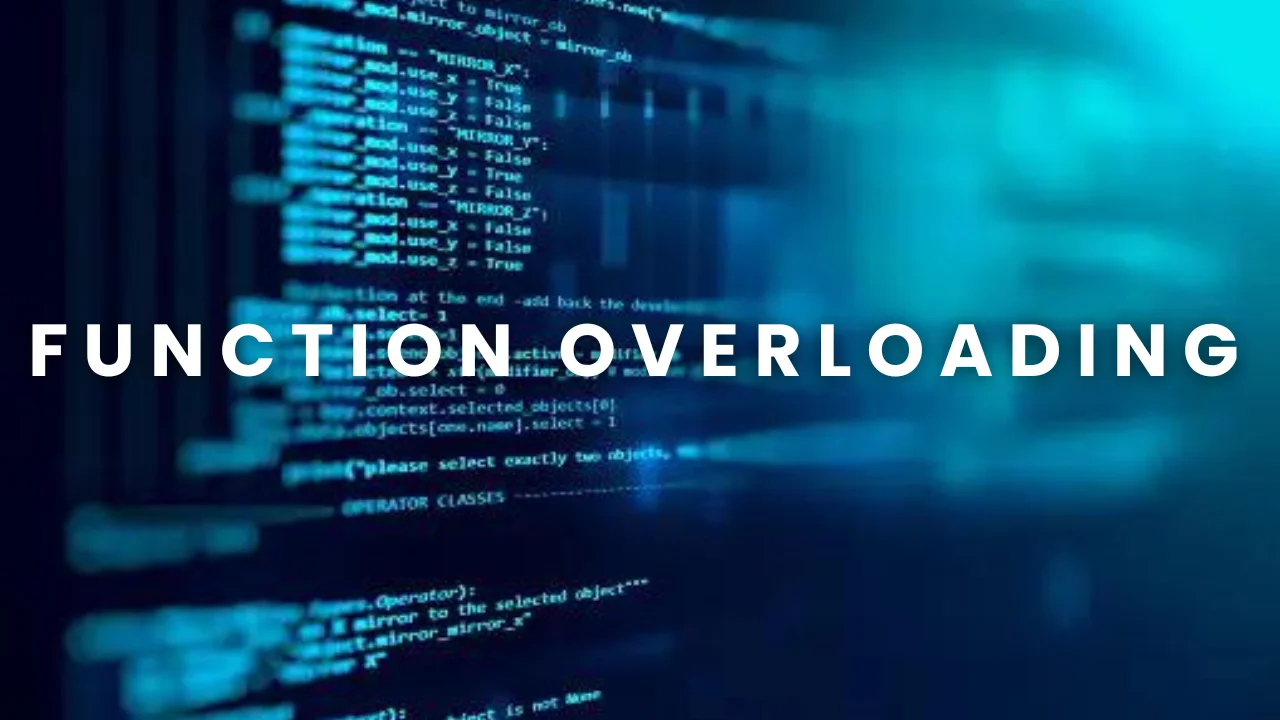
Understanding Method Overloading
Method overloading is a feature in Java that allows a class to have multiple methods with the same name but with different parameters. This provides flexibility in designing classes by allowing developers to create methods that perform similar tasks but with variations in input parameters. Method overloading is based on the concept of polymorphism, where the same method name can behave differently depending on the context of its usage.
// Method definition 1
returnType methodName(parameterType1 param1, parameterType2...) {
// Method body
}
// Method definition 2 (overloaded version)
returnType methodName(parameterType1 param1, parameterType2...) {
// Method body
}
// Additional overloaded methods can be defined similarly
}
1.public class ClassName { ... }: The method overloading syntax is typically defined within a class.
2.Return Type: Specifies the data type of the value that the method returns. Use 'void' if the method does not return any value.
3.Method Name:Should be a valid identifier following Java naming conventions.
4.Parameter List:Specifies the data type and name of each parameter that the method accepts. Multiple parameters are separated by commas.
5.{ ... } This block contains the method body, where the actual functionality of the method is defined..
Question 1. Write a class with name Area using function overloading that computes the area of a parallelogram, a rhombus and a trapezium. Area of a parallelogram (pg) = b*h , Area of a rhombus (rh) = 1/2*d1*d2 (d1 & d2 are the diagonals) , Area of a trapezium (tr) = 1/2*( a + b)* h (where a and b are the parallel sides, h= perpendicular distance the parallel sides)
OUTPUT:
Enter the base and height of the parallelogram:
10
20
The area of a parallelogram is:200
Enter the 1st and 2nd diagonal of a rhombus:
10
5
The area of a rhombus is:25.0
Enter the parallel sides and perpendicular distance the parallel sides of a trapezium:
5
5
10
The area of a trapezium is:50.0
Question 2..Write a class with the name Perimeter using function overloading that computers the perimeter of a square, a rectangle and circle. Perimeter of a square =4*s , Perimeter of a rectangle = 2*(l+b), Perimeter of a circle= 2* π * r.
OUTPUT:
Enter s,l,b,r
10
20
5
15
Perimeter of a square=40.0
Perimeter of a rectangle=50
Perimeter of a circle=681.0
1. Write a program to interchange the value (swap) of the two numbers a and b and display the result after swapping. Use overload function display (int,int) and display(float,float) for swapping integer and float type values.
2. Write a class with the name volume using function overloading that computes the volume of a cube, a sphere and a cuboid. Volume of a cube (vc) = s*s*s , Volume of a sphere (vs) = 4/3* π *r*r*rVolume of a cuboid (vcd) = l*b*h.
In conclusion, method overloading in Java is a powerful feature that promotes code clarity, flexibility, and reusability by allowing methods with the same name but different parameter lists. It facilitates cleaner code organization and contributes to polymorphism, enhancing the overall maintainability of Java programs.