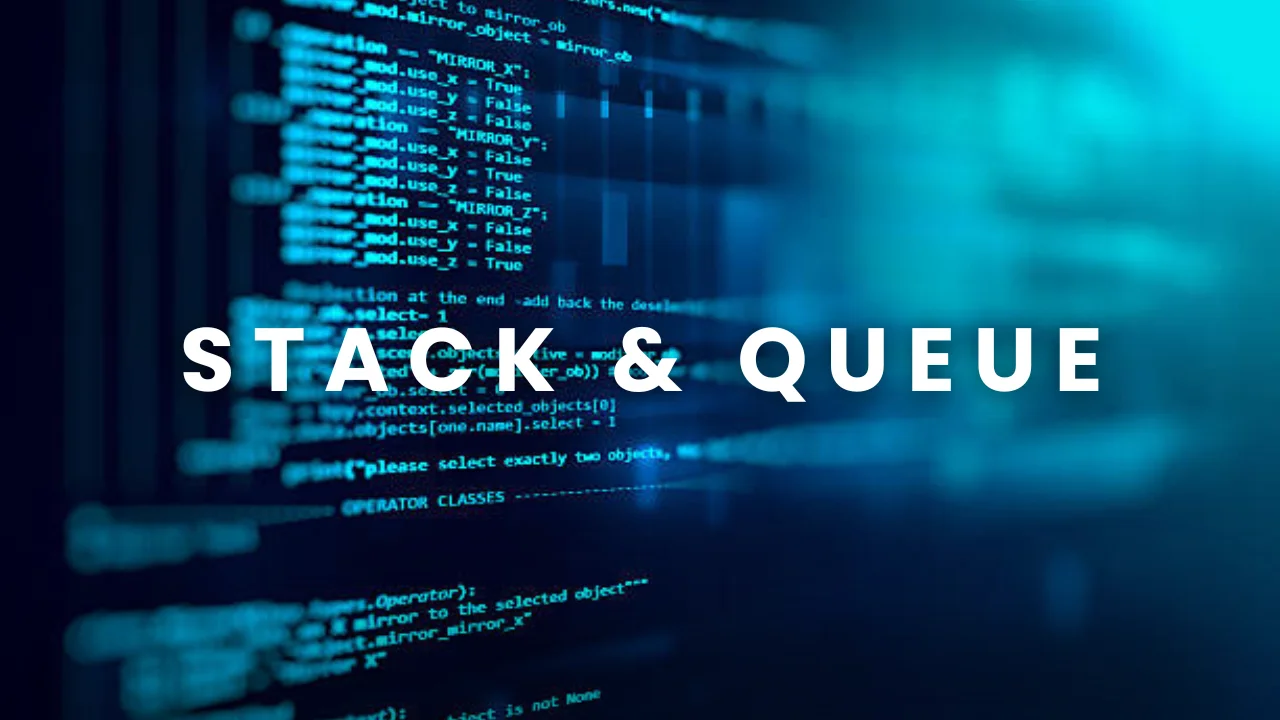
Exploring Stacks & Queues in Python
Stack: Imagine a stack of books where you can only add or remove
books from the top. In programming, a stack is a data structure that follows
the Last-In, First-Out (LIFO) principle. It means the last element added to
the stack will be the first one to be removed.
Queue: Picture a queue of people waiting in line at a ticket counter.
In programming, a queue is a data structure that follows the First-In,
First-Out (FIFO) principle. It means the first element added to the queue
will be the first one to be removed.
Stack
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
Queue:
def __init__(self):
self.items = []
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.pop(0)
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
Stack
push(item): Adds an item to the top of the stack.
pop(): Removes and returns the top item from the stack.
peek(): Returns the top item from the stack without removing it.
is_empty(): Checks if the stack is empty.
size(): Returns the number of items in the stack.
Queue
enqueue(item): Adds an item to the rear of the queue.
dequeue(): Removes and returns the front item from the queue.
is_empty(): Checks if the queue is empty.
size(): Returns the number of items in the queue.
stack = Stack()
# Push items onto the stack
stack.push(10)
stack.push(20)
stack.push(30)
# Pop items from the stack
print(stack.pop()) # Output: 30
print(stack.pop()) # Output: 20
queue = Queue()
# Enqueue items into the queue
queue.enqueue('apple')
queue.enqueue('banana')
queue.enqueue('cherry')
# Dequeue items from the queue
print(queue.dequeue()) # Output: 'apple'
print(queue.dequeue()) # Output: 'banana'
In summary, stacks and queues are fundamental data structures used in computer science and programming. Stacks follow the Last-In, First-Out (LIFO) principle, while queues follow the First-In, First-Out (FIFO) principle. They can be implemented in Python using lists and support various operations for adding, removing, and accessing elements. Stacks and queues are widely used in algorithms, data processing, and simulation tasks.