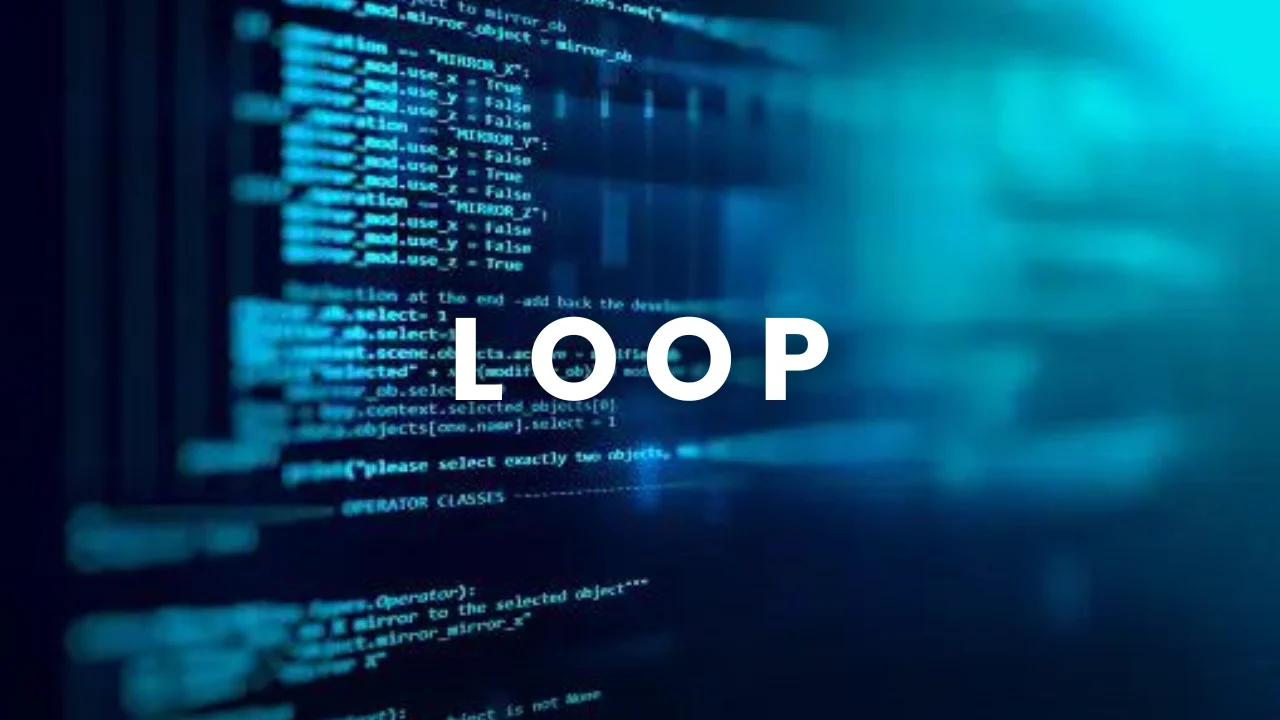
Loops in Paython
Loops are programming structures that repeat a block of code until a certain condition is met. They streamline repetitive tasks, enhancing efficiency and reducing redundancy in code.
Python offers two primary loop constructs:
1. For Loops:This loop iterates over a sequence (such as a list, tuple, string, or range) or any iterable object. It executes a block of code for each element in the sequence.
2. While Loops:This loop repeats a block of code as long as a specified condition is true. It continues execution until the condition becomes false.
3. Nested loops:These are loops within loops. You can have a for loop inside a for loop, a while loop inside a for loop, or vice versa. They are used to execute a set of statements repeatedly in a nested manner.
Iteration lies at the heart of programming, enabling the repetitive execution of a set of instructions. In Python, iteration is achieved through various loop constructs, allowing programmers to efficiently traverse through collections of data.
Fundamental Concept of Iteration in Python:At its core, iteration in Python revolves around the concept of looping
through elements in a sequence or collection. This sequence can be anything
from a list, tuple, string, dictionary, or any other iterable object.
Python provides several loop constructs for iteration, each with its own use
cases and advantages. These constructs include for loops, while loops, and
more specialized constructs like list comprehensions.
Facilitating Iteration over Data Structures:
Loops in Python greatly simplify the process of iterating over data
structures. Whether you're working with lists, tuples, strings, or other
iterable objects, Python's loop constructs offer intuitive mechanisms for
traversal.
For example, with a list my_list, you can easily iterate over its elements
using a for loop:
for item in my_list:
print(item)
Readability: Python's loop constructs are designed for
readability, making
code easy to understand and maintain. The explicit nature of loops enhances
code clarity, especially when dealing with repetitive tasks.
Flexibility: Loops provide flexibility in handling
different data structures
and scenarios. Whether you're iterating over a collection of integers,
strings, or complex objects, Python's loop constructs adapt effortlessly.
Efficiency: Iteration with loops is often more efficient
than manual
iteration, as Python's built-in iteration mechanisms are optimized for
performance. This efficiency is particularly evident when working with large
datasets or complex data structures.
In Python, the range() function is super helpful when you want to create a sequence of numbers. This sequence can be used in loops to repeat certain actions a specific number of times. Let's break it down:
How to use range() in loops:Let's say you want to print numbers from 0 to 4. You can use range() like this:
print(number)
#output : 0 1 2 3 4
If you want to start from a different number, you can tell range() where to begin. For example, to print numbers from 2 to 5:
print(number)
#output : 2 3 4 5