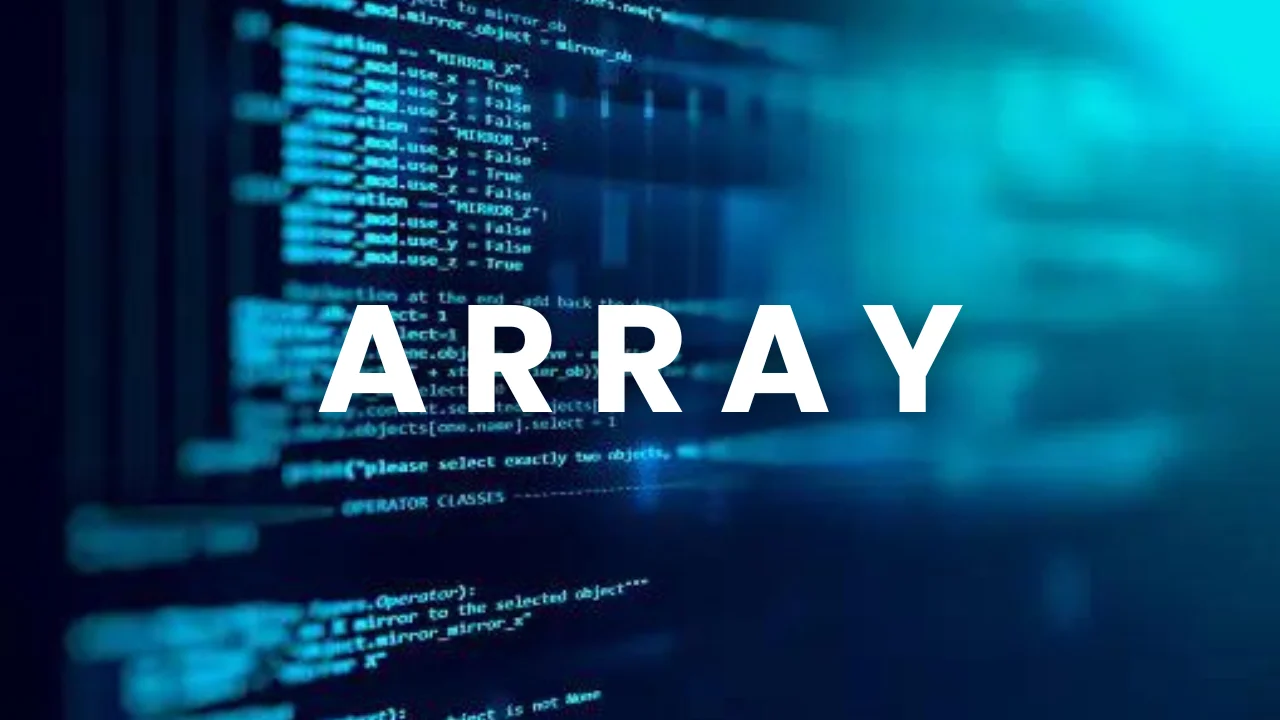
Understanding Arrays in Python
Arrays are fundamental data structures in programming that allow you to store collections of elements. In Python, arrays are implemented using lists, which are versatile and easy to use. Let's explore arrays in Python in a beginner-friendly manner.
An array is a data structure that can hold a fixed-size sequential collection of elements of the same type. Each element in an array is identified by an index. Arrays are commonly used for organizing and manipulating data in programming.
In Python, arrays are typically implemented using lists, which are ordered collections of elements. Here's how you can create an array in Python:
In this example, my_array is an array containing integers from 1 to 5.
You can access individual elements in an array using their index. Indexing in Python starts from 0. Here's how you can access elements in an array:
print(my_array[2]) # Output: 3
You can update the value of an element in an array by assigning a new value to its index. Here's how you can update elements in an array:
print(my_array) # Output: [1, 10, 3, 4, 5]
In this example, the value at index 1 is updated to 10.
Arrays support various operations in Python, such as concatenation, slicing, and finding the length. Here are some common array operations:
array1 = [1, 2, 3]
array2 = [4, 5, 6]
concatenated_array = array1 + array2
print(concatenated_array) # Output: [1, 2, 3, 4, 5, 6]
# Slicing
sliced_array = my_array[1:4]
print(sliced_array) # Output: [10, 3, 4]
# Length
print(len(my_array)) # Output: 5
1. Student Grades Calculation:
You are tasked with calculating the average grade of students in a class.
Create a Python program that stores student grades in an array and
calculates the average grade for the class.
student_grades = [85, 90, 78, 92, 88]
# Function to calculate average grade
def calculate_average_grade(grades):
total = sum(grades)
average = total / len(grades)
return average
# Example usage
average_grade = calculate_average_grade(student_grades)
print("Average grade:", average_grade)
2. Product Sales Analysis:
You need to analyze the sales data for a set of products. Create a Python
program that stores product sales in an array and calculates the total sales
revenue.
product_sales = [1000, 1500, 2000, 1200, 1800]
# Function to calculate total sales revenue
def calculate_total_sales(sales):
total = sum(sales)
return total
# Example usage
total_revenue = calculate_total_sales(product_sales)
print("Total sales revenue:", total_revenue)
3. Temperature Monitoring System:
Develop a temperature monitoring system that tracks temperature readings
throughout the day. Create a Python program that stores temperature readings
in an array and identifies the maximum temperature recorded.
temperature_readings = [22.5, 24.8, 25.3, 21.7, 23.9]
# Function to find maximum temperature
def find_maximum_temperature(temperatures):
maximum_temp = max(temperatures)
return maximum_temp
# Example usage
max_temp = find_maximum_temperature(temperature_readings)
print("Maximum temperature recorded:", max_temp)
4. Polling Data Analysis:
Analyze the results of a survey conducted with multiple-choice questions.
Create a Python program that stores the frequency of responses for each
question in an array and identifies the most common response for each
question.
polling_data = [
[10, 20, 15], # Responses for Question 1
[25, 30, 20] # Responses for Question 2
]
# Function to find most common response for each question
def find_most_common_responses(data):
common_responses = []
for question_responses in data:
most_common_response = max(question_responses)
common_responses.append(most_common_response)
return common_responses
# Example usage
most_common_responses = find_most_common_responses(polling_data)
print("Most common responses for each question:", most_common_responses)
Conclusion:
Arrays are essential data structures in programming that allow you to store
and manipulate collections of elements efficiently. In Python, arrays are
implemented using lists, which offer flexibility and ease of use. By
understanding arrays, you can effectively organize and process data in your
Python programs.