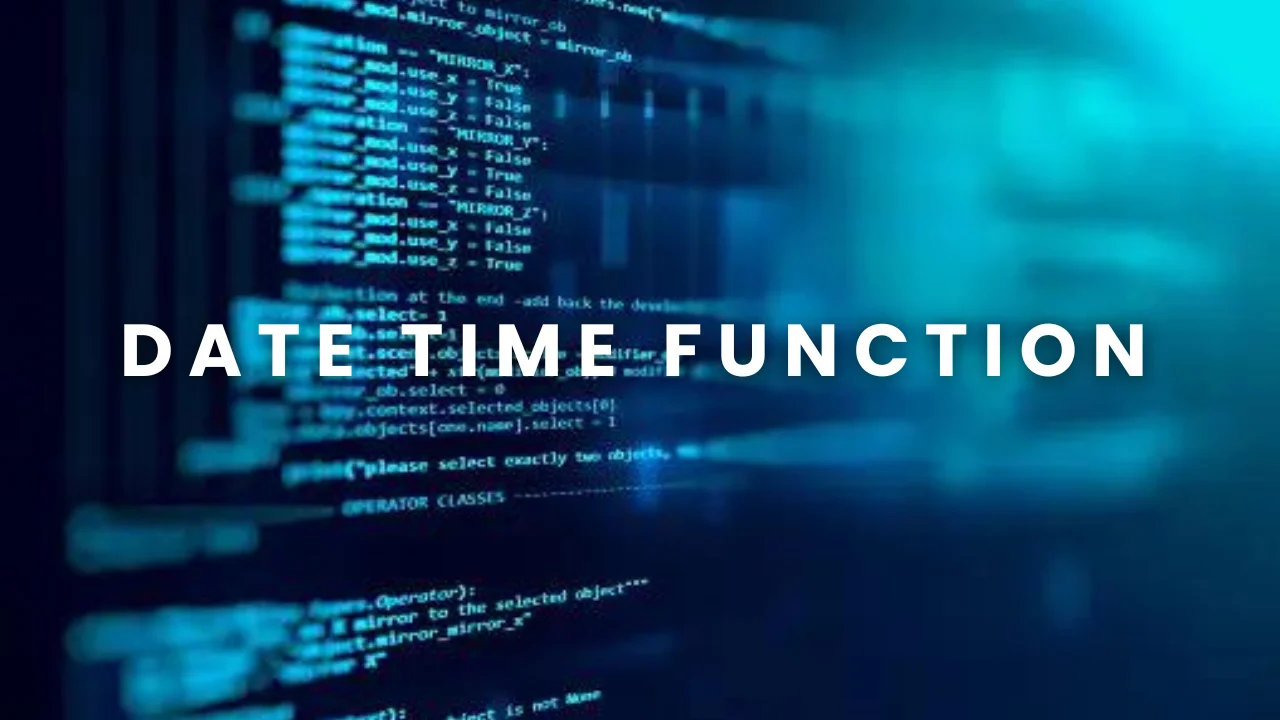
Exploring Date & Time Functions
In Python, the datetime module provides classes for working with dates and times. These classes allow you to manipulate, format, and perform calculations on dates and times effectively. Let's delve into date and time functions in Python in a beginner-friendly manner.
Dates and times are essential components in programming for tasks such as scheduling, logging, and data analysis. In Python, dates represent specific calendar dates, while times represent specific points in time during a day.
The datetime module in Python provides classes for working with dates and times. The primary classes include:
datetime: Represents a specific date and time.
date: Represents a specific date without time information.
time: Represents a specific time without date information.
timedelta: Represents the difference between two dates or times.
The datetime module offers various functions and methods to manipulate and work with dates and times efficiently. Some common functions include:
datetime.now(): Returns the current date and time.
datetime.today(): Returns the current local date and time.
datetime.strptime(): Parses a string representing a date and time into a datetime object.
datetime.strftime(): Formats a datetime object as a string according to a specified format.
datetime.timedelta(): Represents a duration, such as days, seconds, and microseconds, between two datetime objects.
Let's see some examples demonstrating the usage of date and time functions in Python:
import datetime
# Get the current date and time
current_datetime = datetime.datetime.now()
print("Current Date and Time:", current_datetime)
# Parse a string to a datetime object
date_string = "2023-12-31"
parsed_date = datetime.datetime.strptime(date_string, "%Y-%m-%d")
print("Parsed Date:", parsed_date)
# Format a datetime object as a string
formatted_date = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
print("Formatted Date:", formatted_date)
# Calculate the difference between two dates
future_date = parsed_date + datetime.timedelta(days=100)
print("Future Date:", future_date)
Event Scheduler Application:
Q. You are developing an event scheduler application that allows users to
schedule and manage upcoming events, such as meetings, appointments, and
deadlines. Users should be able to view, add, update, and delete events, as
well as receive reminders before scheduled events.
class Event:
def __init__(self, name, date_time):
self.name = name
self.date_time = date_time
def add_event(name, date_time):
event = Event(name, date_time)
# Add event to the database or storage
def get_upcoming_events():
current_datetime = datetime.datetime.now()
# Query database or storage for upcoming events after the current datetime
# Return a list of upcoming events
def send_reminder(event):
# Send reminder notification to the user before the event date
pass
# Example usage
event_name = "Team Meeting"
event_datetime = datetime.datetime(2024, 4, 1, 14, 30) # April 1, 2024, 2:30 PM
add_event(event_name, event_datetime)
upcoming_events = get_upcoming_events()
for event in upcoming_events:
send_reminder(event)
Data Analysis and Reporting:
Q. You are working on a data analysis project that involves processing
time-stamped data and generating reports based on various time intervals,
such as daily, weekly, and monthly summaries. You need to calculate metrics,
such as averages, totals, and counts, for different time periods.
def calculate_daily_metrics(data):
# Calculate daily metrics (e.g., averages, totals) from time-stamped data
# Return daily metrics
def calculate_weekly_metrics(data):
# Calculate weekly metrics (e.g., averages, totals) from time-stamped data
# Return weekly metrics
def calculate_monthly_metrics(data):
# Calculate monthly metrics (e.g., averages, totals) from time-stamped data
# Return monthly metrics
# Example usage
time_series_data = [
{"timestamp": datetime.datetime(2024, 3, 28, 10, 15), "value": 100},
{"timestamp": datetime.datetime(2024, 3, 29, 11, 30), "value": 150},
{"timestamp": datetime.datetime(2024, 3, 30, 9, 45), "value": 120},
# Additional time-stamped data
]
daily_metrics = calculate_daily_metrics(time_series_data)
weekly_metrics = calculate_weekly_metrics(time_series_data)
monthly_metrics = calculate_monthly_metrics(time_series_data)
print("Daily Metrics:", daily_metrics)
print("Weekly Metrics:", weekly_metrics)
print("Monthly Metrics:", monthly_metrics)
String functions in Python provide a wide range of operations to manipulate and work with strings effectively. By using these functions, you can perform tasks such as string formatting, searching, splitting, and modification with ease. Understanding and utilizing string functions are essential skills for working with text data in Python.