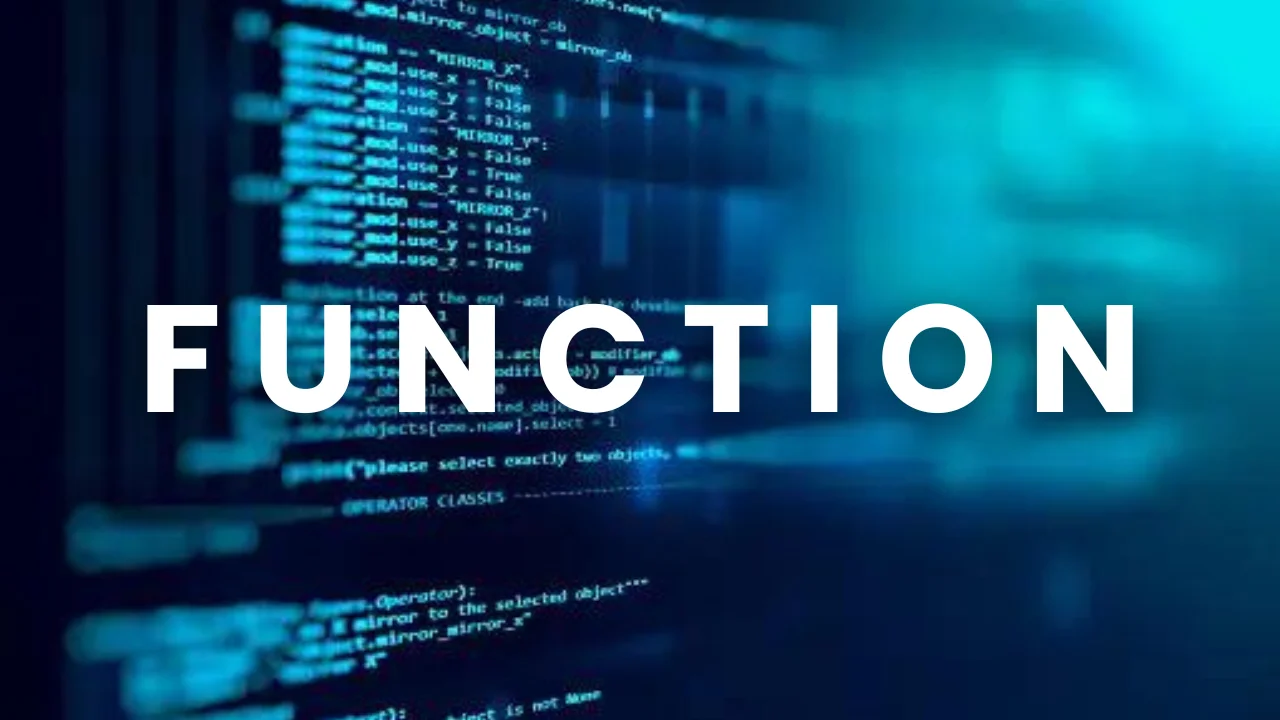
Understanding Python Functions
Introduction:
Python functions are essential building blocks in programming. They allow
you to break down your code into reusable chunks, making it easier to manage
and maintain. In this guide, we'll walk through the basics of Python
functions, covering what they are, how to define them, and how to use them
effectively.
In Python, a function is a block of code that performs a specific task. Functions take input, perform some computation, and return output. They help in organizing code, making it more modular and easier to understand.
To define a function in Python, you use the def keyword followed by the function name and parameters enclosed in parentheses. Here's a simple example:
print("Hello, " + name + "!")
In this example, greet is the name of the function, and name is a parameter that the function takes.
Once you've defined a function, you can call it by using its name followed by parentheses and passing the required arguments. Here's how you call the greet function:
# This will print "Hello, Alice!" to the console.
Functions can return a value using the return statement. Here's an example:
return a + b
In this example, the add function takes two parameters a and b, adds them together, and returns the result.
print(result) # Output: 8
Default Parameters:
You can also specify default values for parameters in a function. These
default values are used when the function is called without providing a
specific argument for that parameter. Here's an example:
print("Hello, " + name + "!")
print("Hello, World!")
# 2. input(): Used to take input from the user.
name = input("Enter your name: ")
# 3. len(): Returns the length of an object (e.g., string, list, tuple).
length = len("Hello")
# 4. type(): Returns the type of an object.
data_type = type(10)
# 5. range(): Generates a sequence of numbers.
numbers = range(1, 5)
# 6. sum(): Calculates the sum of elements in an iterable.
total = sum([1, 2, 3, 4, 5])
# 7. max(): Returns the largest item in an iterable.
largest = max(10, 20, 30)
# 8. min(): Returns the smallest item in an iterable.
smallest = min([5, 2, 8, 1])
# 9. abs(): Returns the absolute value of a number.
absolute_value = abs(-10)
# 10. sorted(): Returns a sorted list of the specified iterable.
sorted_list = sorted([3, 1, 4, 2])
# 11. str(): Converts an object into a string.
string = str(123)
# 12. int(): Converts a string or a number to an integer.
integer = int("123")
# 13. float(): Converts a string or a number to a floating-point number.
floating_point = float("3.14")
# 14. list(): Converts an iterable (e.g., tuple, string) to a list.
my_list = list((1, 2, 3))
# 15. tuple(): Converts an iterable (e.g., list, string) to a tuple.
my_tuple = tuple([1, 2, 3])
now here
Example 1: Calculating the Area of a Rectangle
In this example, we'll create a function to calculate the area of a rectangle based on its width and height.
"""Calculate the area of a rectangle."""
return width * height
# Input values
rectangle_width = 5
rectangle_height = 3
# Calculate the area using the function
area = calculate_rectangle_area(rectangle_width, rectangle_height)
# Output the result
print("The area of the rectangle is:", area)
Example 2: Generating Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1.
"""Generate the Fibonacci sequence up to n terms."""
fib_sequence = []
a, b = 0, 1
for _ in range(n):
fib_sequence.append(a)
a, b = b, a + b
return fib_sequence
# Input value
sequence_length = 10
# Generate the Fibonacci sequence using the function
fibonacci_sequence = generate_fibonacci_sequence(sequence_length)
# Output the result
print("Fibonacci Sequence:", fibonacci_sequence)
Example 3: Temperature Conversion
In this example, we'll create functions to convert temperatures between Celsius and Fahrenheit.
"""Convert temperature from Celsius to Fahrenheit."""
return (celsius * 9/5) + 32
def fahrenheit_to_celsius(fahrenheit):
"""Convert temperature from Fahrenheit to Celsius."""
return (fahrenheit - 32) * 5/9
# Convert Celsius to Fahrenheit
celsius_temperature = 30
fahrenheit_result = celsius_to_fahrenheit(celsius_temperature)
print(f"{celsius_temperature} degrees Celsius is equal to {fahrenheit_result:.2f} degrees Fahrenheit.")
# Convert Fahrenheit to Celsius
fahrenheit_temperature = 86
celsius_result = fahrenheit_to_celsius(fahrenheit_temperature)
print(f"{fahrenheit_temperature} degrees Fahrenheit is equal to {celsius_result:.2f} degrees Celsius.")
Example 4: Shopping Cart Total
In this example, we'll create a function to calculate the total price of items in a shopping cart, including taxes and discounts.
"""Calculate the total price of items in a shopping cart."""
subtotal = sum(items)
total_tax = subtotal * tax_rate
total_discount = subtotal * discount
total_price = subtotal + total_tax - total_discount
return total_price
# Shopping cart items
cart_items = [10, 20, 30, 40]
# Calculate total price with default tax rate and no discount
total_price = calculate_total_price(cart_items)
print("Total price without discount:", total_price)
# Calculate total price with custom tax rate and discount
custom_tax_rate = 0.1
custom_discount = 0.05
total_price_custom = calculate_total_price(cart_items, tax_rate=custom_tax_rate, discount=custom_discount)
print("Total price with custom tax rate and discount:", total_price_custom)
Conclusion:
In conclusion, Python functions are indispensable tools for organizing code,
promoting reusability, and enhancing readability in programming. By
encapsulating specific tasks or operations within functions, developers can
create modular and efficient code that is easier to manage and maintain.
Throughout this guide, we've explored the fundamentals of Python functions,
including their definition, usage, and various features such as parameters,
return statements, default values, and customization options.
We've also showcased real-life examples to illustrate the practical
applications of functions, ranging from simple calculations like area
computation and temperature conversion to more complex tasks such as
shopping cart total calculation. These examples demonstrate how functions
can be leveraged to streamline common tasks and solve real-world problems
effectively.