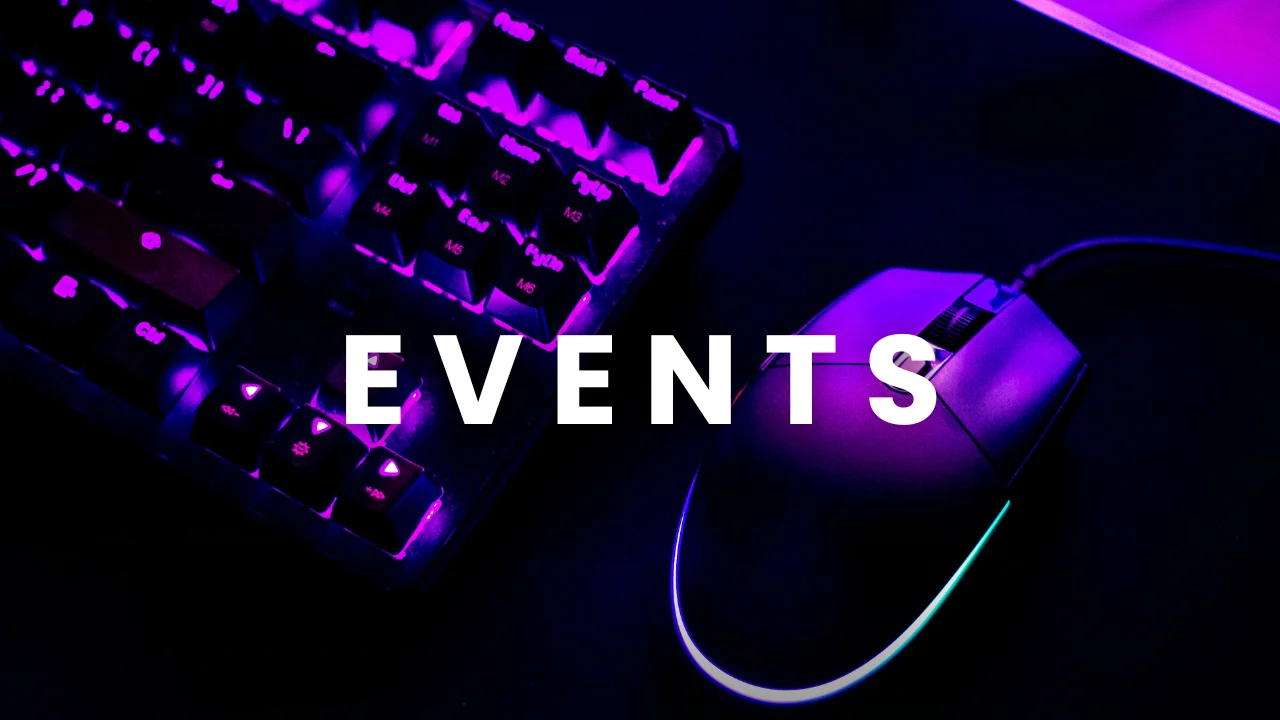
Events in JavaScript
Events in JavaScript are actions or occurrences that happen in the web browser, which can be used to trigger responses in your code. They are a fundamental part of web development, enabling interactive behavior on web pages. Events can be triggered by user actions like clicking, typing, or moving the mouse, as well as by system-generated events like page loading.
Here are some examples of events...
PBA INSTITUTE
Output :
PBA INSTITUTE
Output :
Output :
PBA INSTITUTE
Output :
Understanding events in JavaScript is crucial for creating interactive web applications. By effectively using event listeners, handling event objects, and managing event propagation, you can build dynamic and responsive user interfaces.