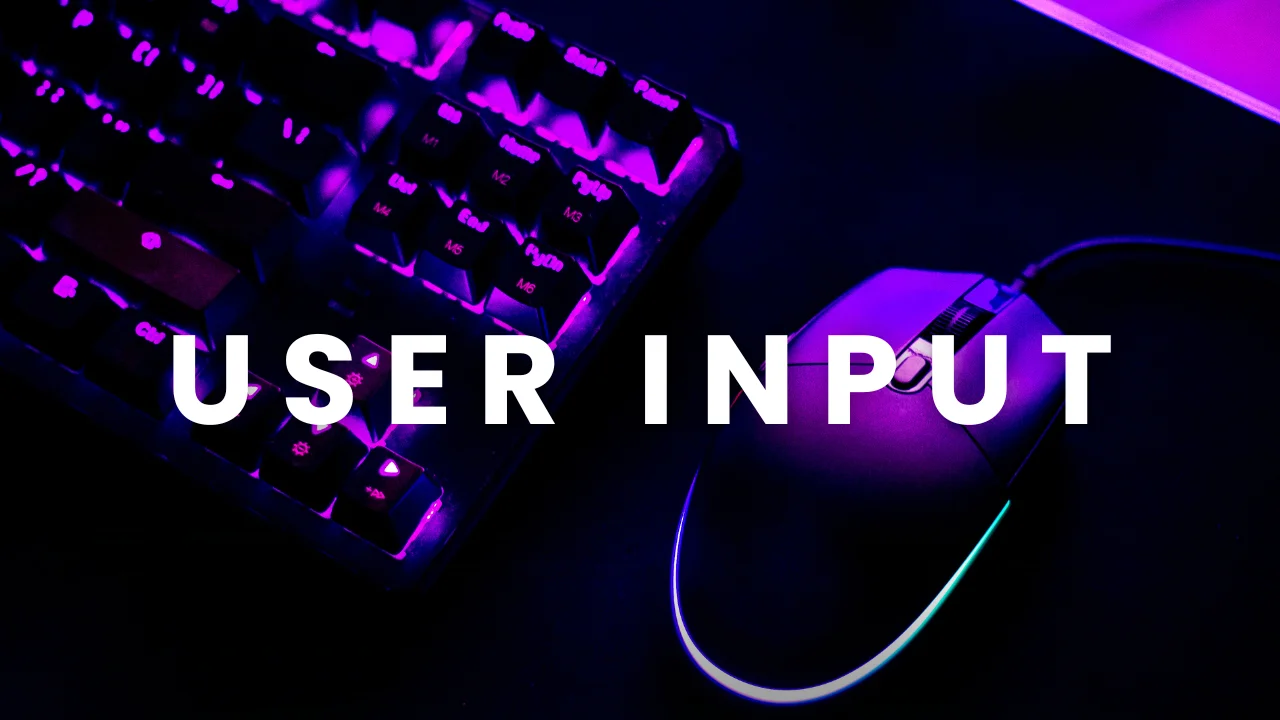
User Input in JavaScript
In this chapter, you'll learn how to interact with your JavaScript programs by taking user input. This allows your programs to be flexible and respond based on what the user provides.
The primary tool for user input in JavaScript is the prompt() function. It pauses your program's execution and waits for the user to type something. Whatever the user types is returned as a string by the prompt() function.
<script type="text/javascript">
var a=prompt("Enter Your Name : ");
</script>
This code displays the prompt "Enter your name: " on the screen. The user types their name and presses Enter. The program then stores the entered name in the variable a.
<script type="text/javascript">
var name=prompt("What is Your Name ? ");
document.write("Hello, "+ name + "!")
</script>
This program asks the user for their name and then prints a personalized greeting.
2. Getting Numbers from the User:<script type="text/javascript">
var age=parseInt(prompt("How old are you?"));
document.write("You are ", age ," years old.");
</script>
Output :
Enter the value of length : 5
Enter the value of width : 5
Area= 25
Perimeter= 20
Diagonal= 7.0710678118654755
BaseArea= 5/2𝑎𝑏, SurfaceArea=5/2ab+5/2bs, Volume=5/6abh. [a – apothem length, b – base length, s – slant height, h – height of the pentagonal pyramid.]
Output :
Enter the value of a :2
Enter the value of b :3
Enter the value of s :4
Enter the value of h :5
Base Area= 15
Surface Area= 30
Volume= 75
Output :
Enter the value of d1 :5
Enter the value of d4 :4
Area=10;
Key Points to Remember:
Practice Exercises:
1. Write a program that asks the user for their favorite food and then prints a message like "Your favorite food is ice cream!".2. Write a program that asks the user for four numbers and then prints their multiplication.
User input is integral to creating dynamic, responsive, and user-centric web applications. It enhances interaction, customization, and functionality, making the web experience more intuitive and engaging.