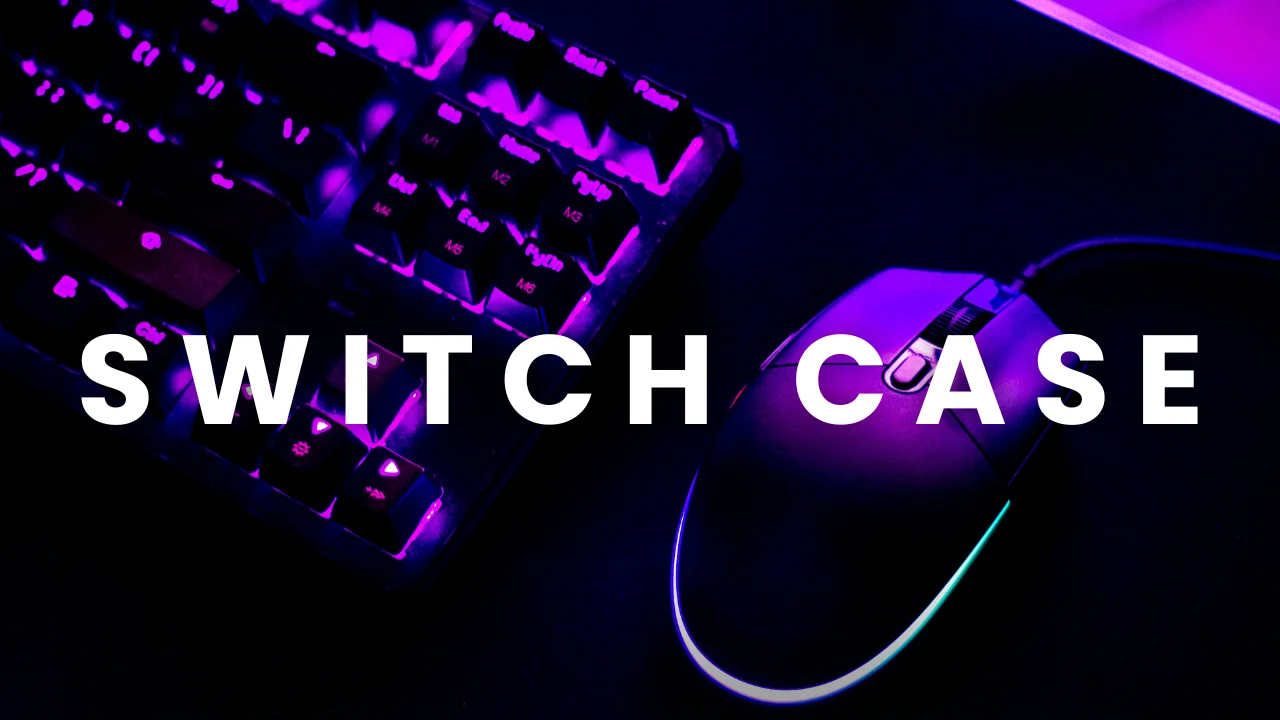
switch case in javascript
A switch statement in JavaScript is a control flow statement used to perform different actions based on different conditions. It evaluates an expression, matching the expression's value to a case clause, and executes the code block associated with that case. If no case matches, an optional default block can be executed. It's commonly used as an alternative to multiple if statements when there are multiple possible conditions to check against a single value.
The structure of a switch statement in JavaScript typically looks like this :
switch (expression) {
case value1:
// Code block to execute if expression === value1
break;
case value2:
// Code block to execute if expression === value2
break;
// Additional cases as needed
default:
// Code block to execute if expression doesn't match any case
}
Here's what each part does:
- switch (expression): This is the expression or variable whose value is being checked against various cases.
- case value1: This is one of the possible values that the expression might have. If the expression is equal to value1, the code block following this case will be executed.
- break;: This keyword is used to exit the switch statement once a case is matched. Without it, execution would continue to the next case, which may not be desired.
- default: This is optional and provides a default case to execute if none of the other cases match the expression.
- // Code block: This is where you put the code that should be executed if the expression matches the corresponding case value.
Remember that each case statement should end with a break statement, unless you intentionally want fall-through behavior (where execution continues to the next case even if the current case matches).
- We have a variable fruit set to "banana".
- We use a switch statement to check the value of fruit.
- Depending on the value of fruit, we set the taste variable accordingly.
- If none of the cases match (for example, if fruit is not "banana", "lemon", "apple", or "orange"), the default case is executed, setting taste to "unknown".
- We print the result, which in this case will be "The taste of the banana is sweet".
Switch case is used in programming to simplify the process of checking multiple possible conditions against a single value. It offers a cleaner and more concise alternative to using multiple if statements.
Here are some reasons why switch case is used:
Readability : It makes the code more readable, especially when there are several conditions to check against one variable.
Efficiency : switch statements can sometimes be more efficient than multiple if statements, especially when there are many conditions to check.
Default case : It allows for a default case to handle situations where none of the conditions match, providing a fallback option.
Ease of Maintenance : It can be easier to maintain and update code using switch statements, especially when adding or removing cases.
Logical Structure : switch statements provide a clear and structured way to handle multiple conditions, improving the organization of the code.
In conclusion, the 'switch' statement is a powerful control flow mechanism in JavaScript that simplifies the process of executing different blocks of code based on the value of an expression. Understanding how to effectively use 'switch' statements can improve code readability and maintainability in JavaScript applications.