
Image Slider
An image slider in CSS is a dynamic component that displays a sequence of images in a slideshow format. It utilizes CSS for layout, styling, and animations, typically combined with JavaScript for interactivity. Key features include transitions between images, navigation controls (such as next and previous buttons), and often responsive design to adapt to different screen sizes. This versatile UI element enhances user experience by showcasing multiple images in a compact, engaging manner within web pages.
OUTPUT:
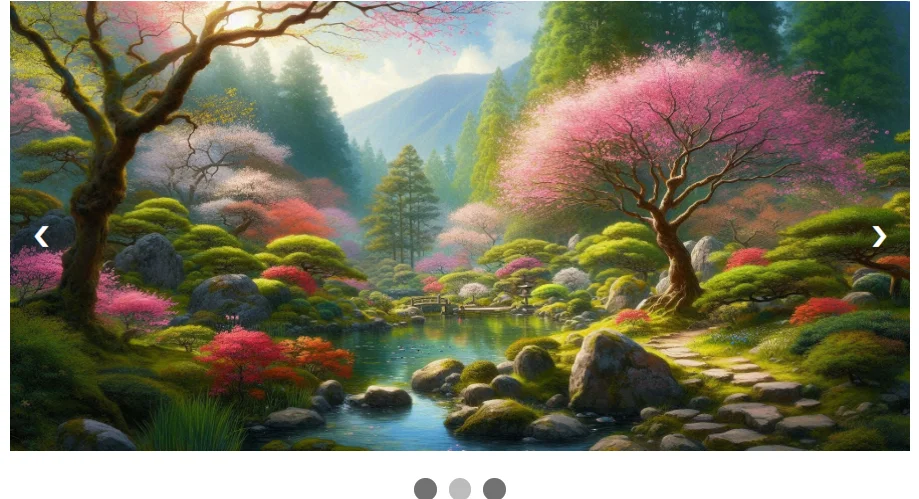
In this example, HTML and CSS code creates an image slideshow. It uses CSS for styling, including buttons for navigation, dots for slide indicators, and responsive adjustments. JavaScript is employed to handle slide transitions and dot activation. Images are displayed in a container with fading transitions between slides, enhancing user interaction and visual appeal on webpages.
In conclusion, CSS image sliders provide a visually appealing and interactive way to showcase content on webpages. They enhance user experience by allowing seamless transitions between images or information, improving engagement and navigation. Lightweight and customizable with CSS, these sliders offer efficient loading times and responsive design, adapting well to various screen sizes and devices. Overall, CSS image sliders are a versatile tool for creating dynamic and engaging web content.