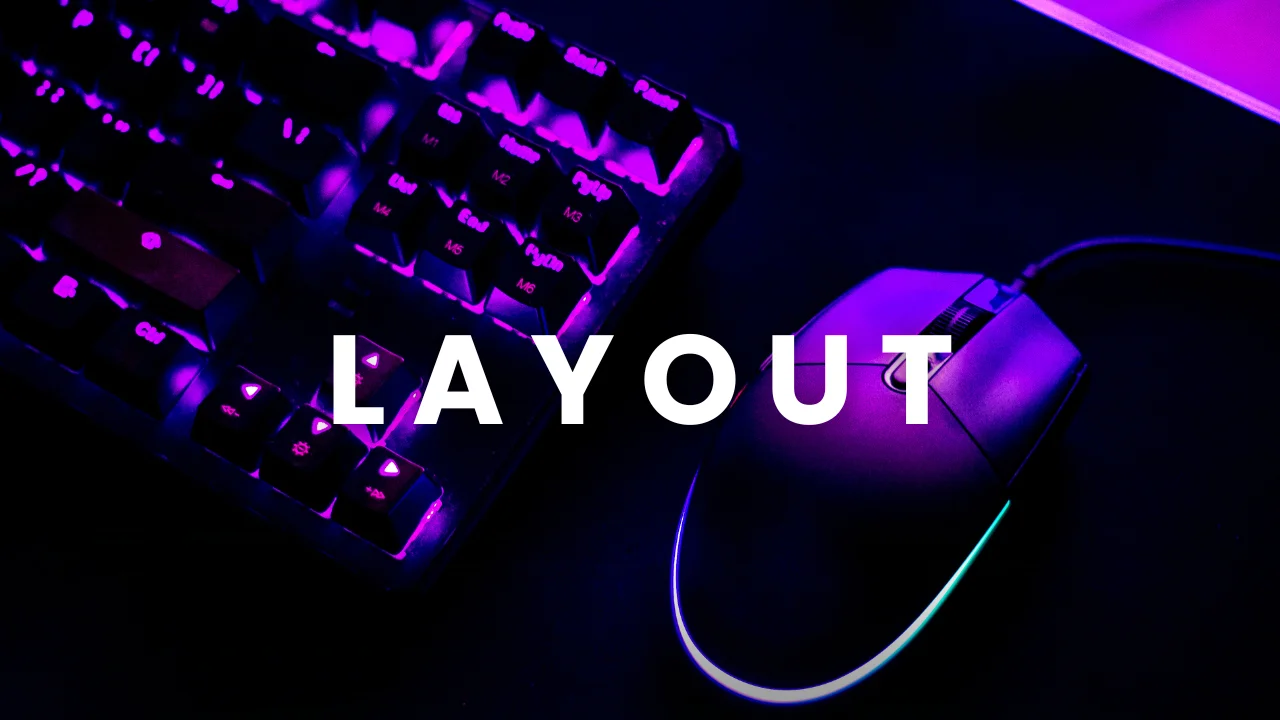
Layout
In CSS, layout refers to how elements are structured and positioned on a webpage. It involves using techniques like floats, flexbox, or CSS Grid to arrange content in columns, rows, or other configurations. Layouts ensure content is visually organized and responsive across different screen sizes. Effective layout design enhances user experience by improving readability, accessibility, and overall aesthetic appeal of websites.
Header
Main Content
This is the main content of the page.
OUTPUT:
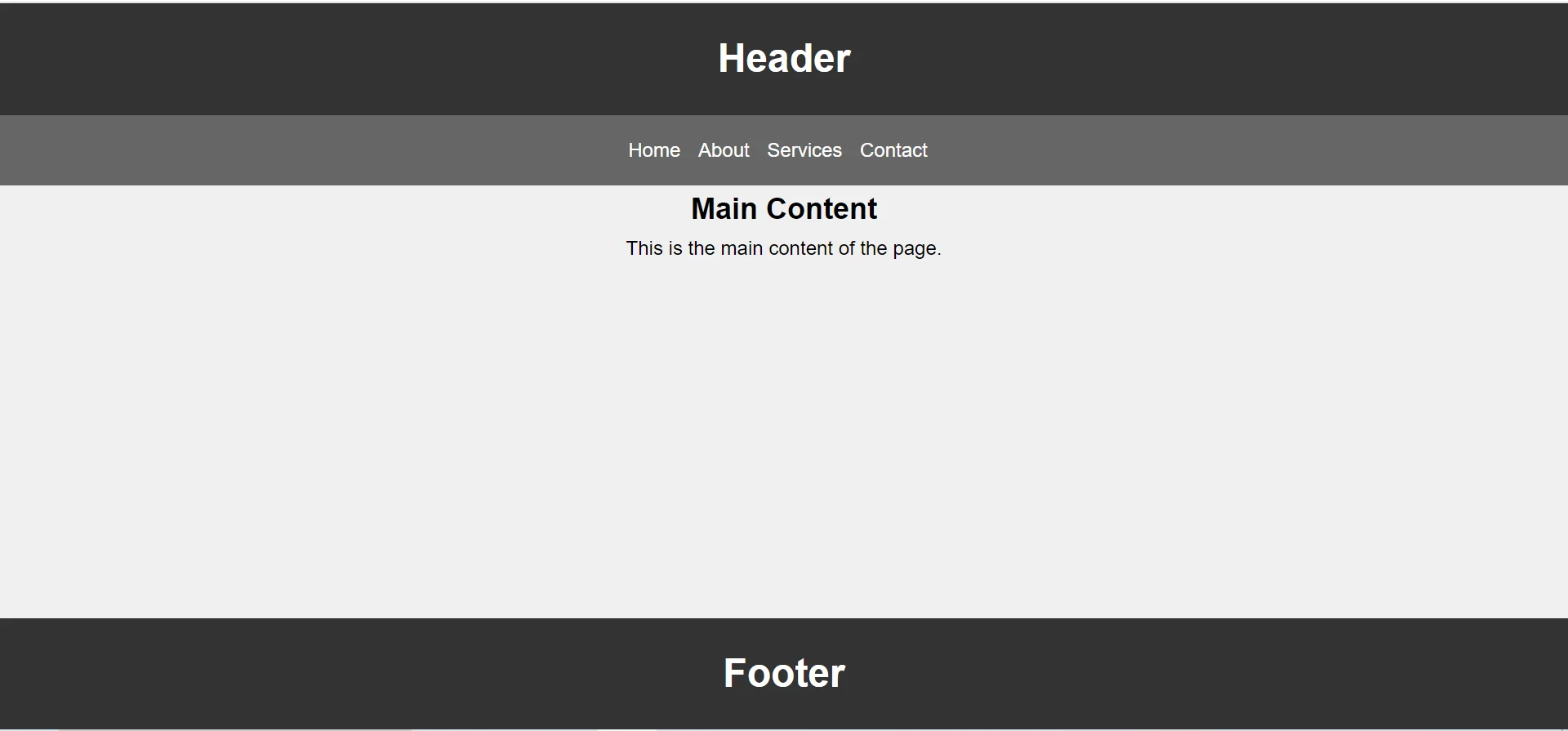
Welcome to PBA INSTITUTE
Example of a Layout.
OUTPUT:
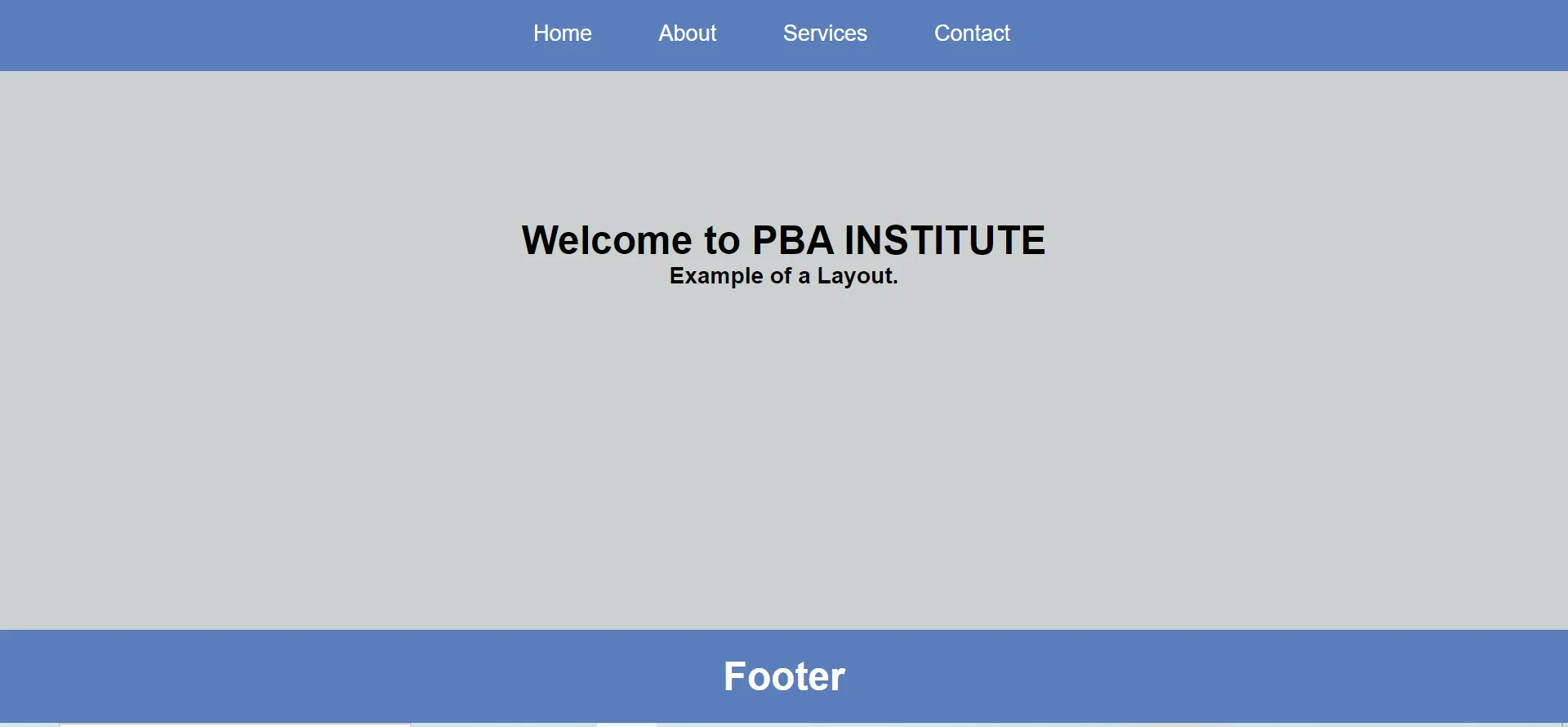
In conclusion, CSS layout offers flexible and precise control over webpage structure, enhancing usability and aesthetics. It enables responsive design, ensuring content adapts seamlessly across devices. CSS Grid and Flexbox simplify complex layouts, improving code maintainability and developer efficiency. Floats provide legacy support for basic layout needs. Overall, CSS layout empowers designers to create visually appealing, accessible, and user-friendly websites, optimizing user experience by organizing content logically and efficiently on both desktop and mobile platforms.